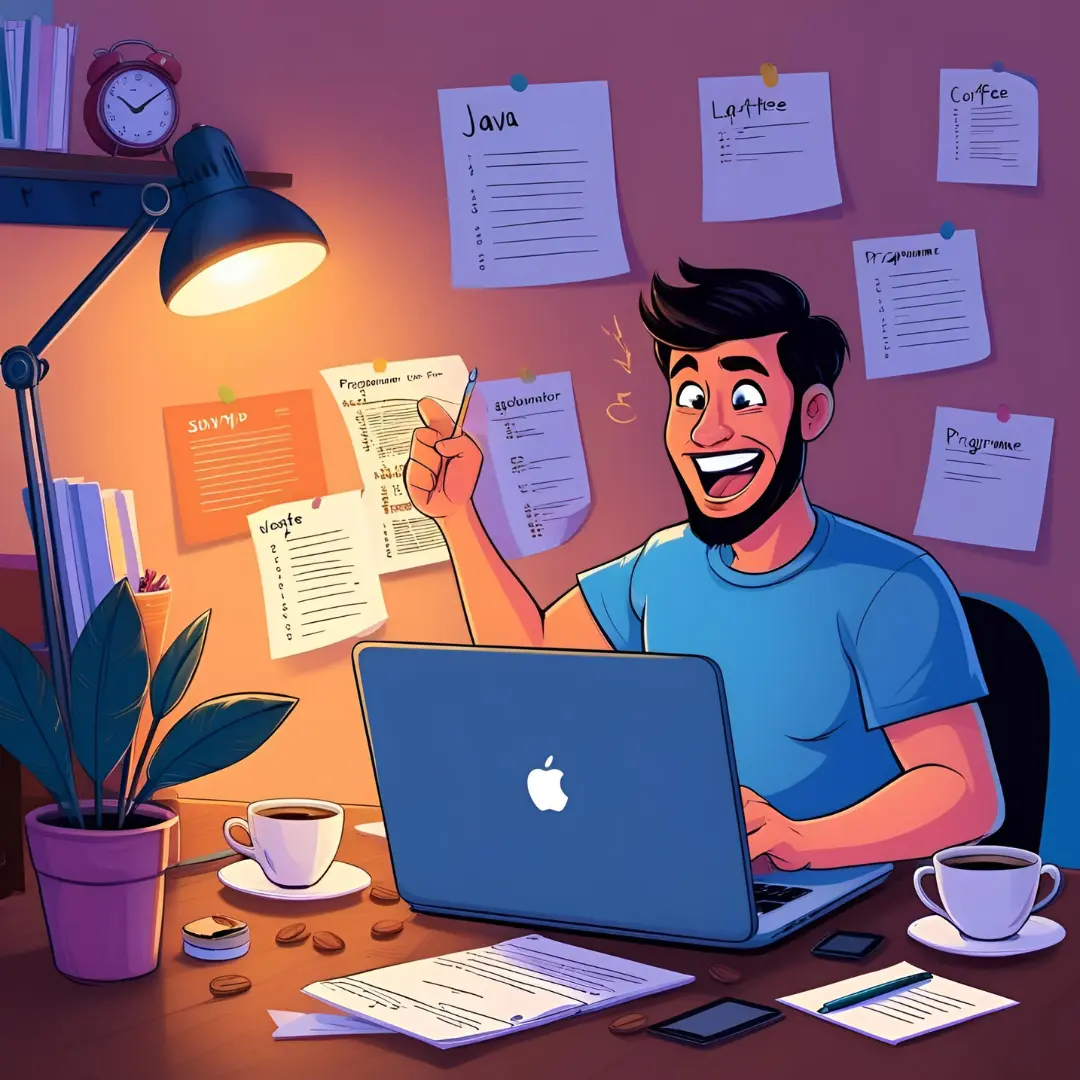
Java 9 introduced new methods in the Stream API to improve functionality and efficiency. These enhancements include takeWhile()
, dropWhile()
, ofNullable()
, and iterate()
improvements.
1. takeWhile(Predicate<T>)
β Take Elements While a Condition is True
This method takes elements from the stream until the predicate becomes false
. Once the condition fails for the first time, it stops processing the stream.
π Example: Using takeWhile()
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 10, 4, 5);
numbers.stream()
.takeWhile(n -> n < 5) // Stops at first number >= 5 (10)
.forEach(System.out::println);
}
}
β Output:
1
2
3
β Once 10
appears (not < 5
), takeWhile()
stops processing the stream.
2. dropWhile(Predicate<T>)
β Drop Elements While a Condition is True
This method drops elements from the stream until the predicate becomes false
. Once a value fails the condition, all remaining elements are included.
π Example: Using dropWhile()
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 10, 4, 5);
numbers.stream()
.dropWhile(n -> n < 5) // Drops 1, 2, 3 but keeps 10, 4, 5
.forEach(System.out::println);
}
}
β Output:
10
4
5
β Drops numbers < 5
but keeps everything from the first 10
onward.
3. Stream.ofNullable(T value)
β Handle Null Values Safely
Previously, Stream.of(null)
threw a NullPointerException
. Now, Stream.ofNullable()
returns an empty stream if the value is null, avoiding errors.
π Example: Handling Nullable Values in Streams
import java.util.stream.Stream;
public class Main {
public static void main(String[] args) {
String nullableValue = null;
// Instead of throwing NullPointerException, returns an empty stream
Stream.ofNullable(nullableValue)
.forEach(System.out::println); // β
No error, prints nothing
}
}
β Output:
(nothing)
β Prevents NullPointerException
when dealing with possibly null
values.
4. Stream.iterate()
Enhancement β Add Stop Condition
Before Java 9, Stream.iterate()
required a manual limit()
, making infinite streams difficult to control.
Now, we can add a stop condition directly inside iterate()
.
π Example: Generating Numbers Using iterate()
import java.util.stream.Stream;
public class Main {
public static void main(String[] args) {
Stream.iterate(1, n -> n < 10, n -> n + 2) // Stops at 9
.forEach(System.out::println);
}
}
β Output:
1
3
5
7
9
β iterate()
now has a built-in stop condition (n < 10
), making it safer.
π Summary of Java 9+ Stream Enhancements
Method | Purpose | Example Usage |
---|---|---|
takeWhile(Predicate<T>) | Takes elements while the condition is true | .takeWhile(n -> n < 5) |
dropWhile(Predicate<T>) | Drops elements while the condition is true | .dropWhile(n -> n < 5) |
ofNullable(T value) | Creates a stream with 0 or 1 element, avoiding null errors | Stream.ofNullable(null) |
iterate(seed, hasNext, next) | Generates a stream with a stop condition | Stream.iterate(1, n -> n < 10, n -> n + 2) |
Lesson Reflection
- How does
takeWhile()
improve performance compared tofilter()
? - When would
Stream.ofNullable()
be useful in a real-world scenario? - Why is the Java 9+ version of
iterate()
better than the Java 8 version?
Answers to Reflection Questions on Java 9+ Stream Enhancements
1. How does takeWhile()
improve performance compared to filter()
?
β
takeWhile()
is more efficient than filter()
for sorted streams because it stops processing as soon as the condition fails, while filter()
checks every element in the stream.
π Example: takeWhile()
vs. filter()
Using takeWhile()
(Stops Early)
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 10, 4, 5);
numbers.stream()
.takeWhile(n -> n < 5) // Stops at 10
.forEach(System.out::println);
}
}
β Output:
1
2
3
β Stops at the first 10
(first failure), avoiding unnecessary checks.
Using filter()
(Processes All Elements)
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 10, 4, 5);
numbers.stream()
.filter(n -> n < 5) // Checks all elements, even after first failure
.forEach(System.out::println);
}
}
β Output:
1
2
3
4
5
π¨ Problem: Even though 10
breaks the condition, filter()
keeps checking all elements, reducing efficiency.
β
Key Takeaway:
β Use takeWhile()
when the stream is sorted and elements that fail the condition wonβt be useful.
β Use filter()
when filtering needs to be applied across all elements, regardless of order.
2. When would Stream.ofNullable()
be useful in a real-world scenario?
β
Stream.ofNullable()
prevents NullPointerException
(NPE) when handling possibly null
values.
π Example: Avoiding NullPointerException
in Database Queries
Imagine a function that fetches a username which might be null
:
Without Stream.ofNullable()
(Prone to NPE)
import java.util.stream.Stream;
public class Main {
public static void main(String[] args) {
String username = getUserFromDatabase(); // Could be null
Stream.of(username) // π¨ Throws NullPointerException if username is null
.forEach(System.out::println);
}
static String getUserFromDatabase() {
return null; // Simulating no user found
}
}
π¨ Problem:
Exception in thread "main" java.lang.NullPointerException
Solution: Use Stream.ofNullable()
, which handles null
safely.
β
Using Stream.ofNullable()
(Safe)
import java.util.stream.Stream;
public class Main {
public static void main(String[] args) {
String username = getUserFromDatabase(); // Could be null
Stream.ofNullable(username) // β
Safe, produces an empty stream if null
.forEach(System.out::println);
}
static String getUserFromDatabase() {
return null; // Simulating no user found
}
}
β Output:
(nothing printed, but no error)
β No NullPointerException
, just an empty stream.
β
Key Takeaway:
β Use Stream.ofNullable()
when handling optional values that might be null
to prevent errors.
β Useful for database lookups, API responses, and nullable fields.
3. Why is the Java 9+ version of iterate()
better than the Java 8 version?
β
Java 9+ Stream.iterate()
improves efficiency by including a stop condition, preventing infinite streams when unnecessary.
π Example: Java 8 iterate()
(Requires limit()
)
import java.util.stream.Stream;
public class Main {
public static void main(String[] args) {
Stream.iterate(1, n -> n + 2) // π¨ Infinite if limit() is missing!
.limit(5)
.forEach(System.out::println);
}
}
β Output:
1
3
5
7
9
π¨ Problem:
- If you forget
limit()
, it runs forever! - You must remember to manually add
limit(n)
.
π Java 9+ iterate()
(Built-in Stop Condition)
import java.util.stream.Stream;
public class Main {
public static void main(String[] args) {
Stream.iterate(1, n -> n < 10, n -> n + 2) // β
Stops when n >= 10
.forEach(System.out::println);
}
}
β Output:
1
3
5
7
9
β Automatically stops when n < 10
fails, no limit()
needed.
β
Key Takeaway:
β Java 9+ iterate()
is safer because it has a built-in stop condition, preventing infinite loops.
β Java 8 required limit()
, which was easy to forget.
π Summary
Feature | Java 8 | Java 9+ |
---|---|---|
Stopping condition in iterate() | β Requires limit(n) | β Stops automatically with condition |
Efficient filtering | β filter() checks all elements | β
takeWhile() stops early |
Handling nulls in streams | β Stream.of(null) throws error | β
Stream.ofNullable(null) prevents NPE |
Final Thoughts
β Use takeWhile()
instead of filter()
when possible for better performance.
β Use Stream.ofNullable()
to avoid NullPointerException
when handling nullable data.
β Prefer Java 9+ iterate()
over Java 8βs version to prevent infinite loops.
The next Java 9+ feature: Factory Methods for Collections (List.of()
, Set.of()
, Map.of()
)? π