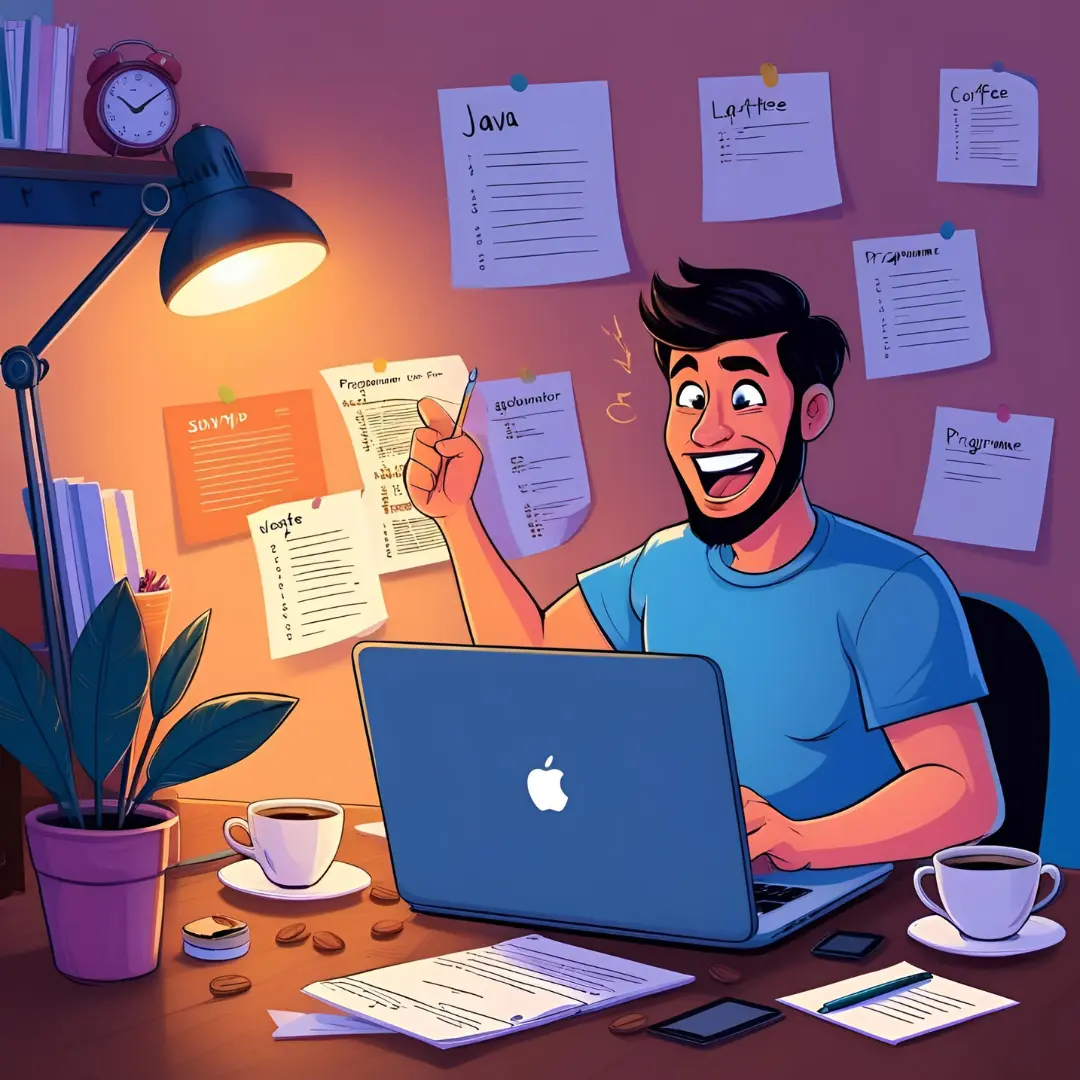
Before Java 8, handling null
values was a common source of bugs and NullPointerException (NPE)
errors. Java 8 introduced Optional<T>
, a container object that may or may not contain a value, to help avoid null
checks.
1. What is Optional
and Why Use It?
Prevents
NullPointerException
(NPE) Encourages better handling of missing values
Makes the intent of “optional” values explicit
Provides cleaner code by reducing explicit
null
checks
2. Creating an Optional
Object
There are three main ways to create an Optional
object:
Method | Description | Example |
---|---|---|
Optional.of(T value) | Creates an Optional with a non-null value. Throws an error if null is passed. | Optional.of("Hello") |
Optional.ofNullable(T value) | Creates an Optional , but allows null . Returns Optional.empty() if null is passed. | Optional.ofNullable(null) |
Optional.empty() | Creates an empty Optional . | Optional.empty() |
Example: Creating an Optional
import java.util.Optional;
public class Main {
public static void main(String[] args) {
Optional<String> nonEmptyOptional = Optional.of("Hello, Java!"); //
Works
Optional<String> nullableOptional = Optional.ofNullable(null); //
Allows null
Optional<String> emptyOptional = Optional.empty(); //
Creates empty Optional
System.out.println("Non-Empty Optional: " + nonEmptyOptional);
System.out.println("Nullable Optional: " + nullableOptional);
System.out.println("Empty Optional: " + emptyOptional);
}
}
Output:
Non-Empty Optional: Optional[Hello, Java!]
Nullable Optional: Optional.empty
Empty Optional: Optional.empty
Optional.empty()
is used instead of null
, making code safer.
3. Checking if an Optional
Has a Value
Method | Description | Example |
---|---|---|
isPresent() | Returns true if there is a value, false otherwise. | opt.isPresent() |
isEmpty() (Java 11+) | Returns true if empty, false otherwise. | opt.isEmpty() |
Example: Checking if an Optional
Has a Value
import java.util.Optional;
public class Main {
public static void main(String[] args) {
Optional<String> opt = Optional.ofNullable(null);
if (opt.isPresent()) {
System.out.println("Value: " + opt.get());
} else {
System.out.println("No value present.");
}
}
}
Output:
No value present.
Avoids
NullPointerException
by checking before accessing the value.
4. Retrieving a Value from Optional
Method | Description | Example |
---|---|---|
get() | Returns the value if present, else throws an exception. | opt.get() |
orElse(T other) | Returns the value, or a default if empty. | opt.orElse("Default Value") |
orElseGet(Supplier<T>) | Returns the value, or a computed value if empty. | opt.orElseGet(() -> "Generated Value") |
orElseThrow(Supplier<Exception>) | Returns the value, or throws an exception if empty. | opt.orElseThrow(() -> new RuntimeException("No value!")) |
Example: Using orElse()
and orElseGet()
import java.util.Optional;
public class Main {
public static void main(String[] args) {
Optional<String> opt = Optional.ofNullable(null);
// Using orElse() - Returns "Default Value" if empty
String result1 = opt.orElse("Default Value");
// Using orElseGet() - Generates a value dynamically if empty
String result2 = opt.orElseGet(() -> "Computed Value");
System.out.println("orElse(): " + result1);
System.out.println("orElseGet(): " + result2);
}
}
Output:
orElse(): Default Value
orElseGet(): Computed Value
orElseGet()
is useful when the default value is expensive to compute, since it only runs if needed.
5. Using ifPresent()
for Clean Code
Instead of checking with isPresent()
, use ifPresent(Consumer<T>)
to execute code only if a value is present.
Example: Using ifPresent()
import java.util.Optional;
public class Main {
public static void main(String[] args) {
Optional<String> opt = Optional.ofNullable("Hello");
opt.ifPresent(value -> System.out.println("Value: " + value));
}
}
Output:
Value: Hello
No need for explicit
if
conditions!
6. Transforming an Optional
Value Using map()
and flatMap()
Method | Description | Example |
---|---|---|
map(Function<T, R>) | Transforms the value if present. | opt.map(String::toUpperCase) |
flatMap(Function<T, Optional<R>>) | Transforms but expects an Optional as output. | opt.flatMap(str -> Optional.of(str.length())) |
Example: Using map()
to Transform an Optional
import java.util.Optional;
public class Main {
public static void main(String[] args) {
Optional<String> opt = Optional.of("hello");
// Convert to uppercase using map()
Optional<String> upperOpt = opt.map(String::toUpperCase);
System.out.println(upperOpt.orElse("No value"));
}
}
Output:
HELLO
map()
transforms only if the value is present.
7. Filtering an Optional
Value
We can use filter(Predicate<T>)
to keep a value only if it matches a condition.
Example: Filtering an Optional
Value
import java.util.Optional;
public class Main {
public static void main(String[] args) {
Optional<String> opt = Optional.of("Java 8");
// Keep value only if it contains "Java"
opt = opt.filter(str -> str.contains("Java"));
System.out.println(opt.orElse("Filtered out"));
}
}
Output:
Java 8
filter()
removes values that don’t match the condition.
Lesson Reflection
- How does
Optional
improve handling ofnull
values? - Why is
orElseGet()
sometimes better thanorElse()
? - Can you think of a real-world example where
Optional
would be useful?
Answers to Reflection Questions on Optional
1. How does Optional
improve handling of null
values?
Prevents
NullPointerException
(NPE)
- Instead of calling a method on
null
and getting an NPE,Optional
forces you to handle the absence of a value safely.
Encourages explicit handling of missing values
Optional
makes it clear in the code when a value might be missing, rather than relying on implicitnull
checks.
Reduces boilerplate
if-else
checks
- Instead of writing multiple
if (obj != null)
checks, you can useorElse()
,orElseGet()
, orifPresent()
.
Functional & Declarative approach
- It integrates well with streams and functional programming (
map()
,filter()
, etc.), making the code cleaner.
2. Why is orElseGet()
sometimes better than orElse()
?
orElse()
always evaluates the default value, even if the Optional
contains a value.orElseGet()
only evaluates when needed, making it more efficient.
Example: Performance Difference Between orElse()
and orElseGet()
import java.util.Optional;
public class Main {
public static void main(String[] args) {
Optional<String> opt = Optional.of("Hello");
System.out.println("Using orElse():");
String result1 = opt.orElse(expensiveComputation()); //
Always runs expensiveComputation()
System.out.println("Using orElseGet():");
String result2 = opt.orElseGet(() -> expensiveComputation()); //
Runs only if Optional is empty
}
public static String expensiveComputation() {
System.out.println("Computing expensive value...");
return "Expensive Value";
}
}
Output:
Using orElse():
Computing expensive value...
Using orElseGet():
Notice that
orElse()
executes expensiveComputation()
even though the Optional
contains a value!
orElseGet()
avoids unnecessary computation because it only runs if the Optional
is empty.
3. Can you think of a real-world example where Optional
would be useful?
Example 1: Avoiding NullPointerException
in a User Database Lookup
In a user authentication system, retrieving a user by ID may return null if the user does not exist.
import java.util.Optional;
class User {
String name;
User(String name) { this.name = name; }
String getName() { return name; }
}
class UserRepository {
static Optional<User> findUserById(int id) {
if (id == 1) return Optional.of(new User("Alice"));
return Optional.empty(); // No user found
}
}
public class Main {
public static void main(String[] args) {
Optional<User> user = UserRepository.findUserById(2); // No user found
// Instead of checking for null, use Optional
String name = user.map(User::getName).orElse("Guest");
System.out.println("User: " + name);
}
}
Output:
User: Guest
If no user is found, we return
"Guest"
instead of crashing with a NullPointerException
.
Example 2: Using ifPresent()
for Logging
import java.util.Optional;
public class Main {
public static void main(String[] args) {
Optional<String> message = Optional.ofNullable(null); // No message
// Log only if there is a message
message.ifPresent(msg -> System.out.println("Logging: " + msg));
}
}
Output:
No log message is printed because the value is absent.
When Should We Prefer orElse()
Over orElseGet()
in Real Projects?
Scenario | Prefer orElse() | Prefer orElseGet() |
---|---|---|
Constant or static default values | ||
Simple calculations | ||
Expensive computations (e.g., complex logic, API calls, DB queries) | ||
Fetching data from an external service | ||
Reading files or large objects | ||
Logging or debugging messages |
Summary:
Use
orElse()
for simple, constant, or static fallback values. Use
orElseGet()
for expensive computations, external service calls, or database queries. If in doubt,
orElseGet()
is usually safer for performance.
Key Takeaways
Optional
makes handling missing values explicit and safe. Use
orElseGet()
instead of orElse()
for better performance when computing default values.
Optional
is ideal for database lookups, API responses, and nullable fields.
next Java 8 feature: Collectors & Method References