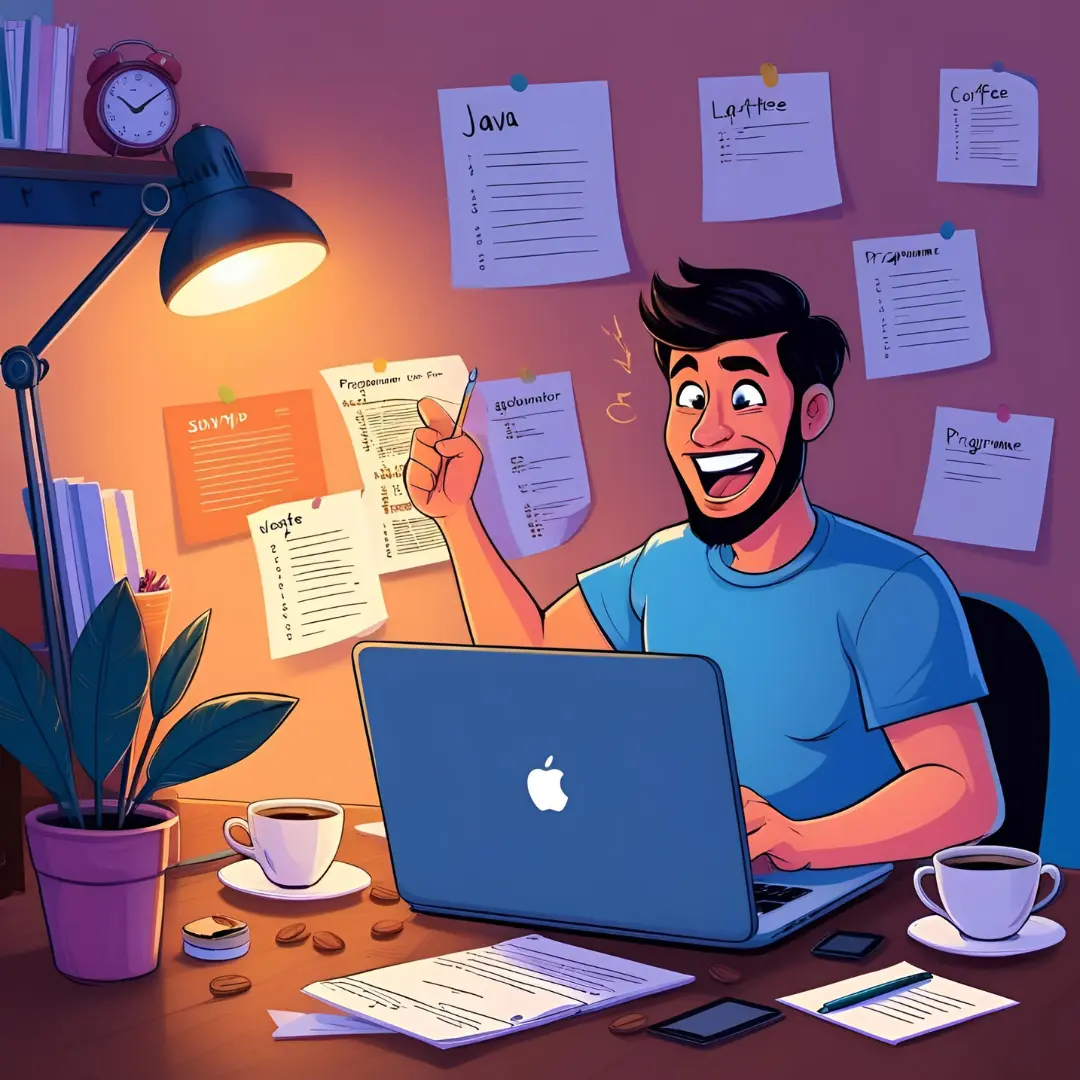
Before Java 8, date and time handling in Java was done using java.util.Date
and java.util.Calendar
, which had several problems:
❌ Mutable objects – Modifying a Date
object would change the original instance.
❌ Thread safety issues – SimpleDateFormat
was not thread-safe.
❌ Confusing APIs – Working with time zones and date calculations was complex.
Java 8 introduced the java.time
package, which provides a more robust, immutable, and easy-to-use Date & Time API.
1. Key Classes in java.time
Package
Class | Description | Example |
---|---|---|
LocalDate | Represents a date (year, month, day) | LocalDate.now() → 2025-02-10 |
LocalTime | Represents a time (hours, minutes, seconds) | LocalTime.now() → 14:30:15.123 |
LocalDateTime | Represents date and time | LocalDateTime.now() → 2025-02-10T14:30:15.123 |
ZonedDateTime | Represents date-time with a time zone | ZonedDateTime.now(ZoneId.of("Asia/Tokyo")) |
Instant | Represents a timestamp (time since Unix epoch) | Instant.now() → 2025-02-10T05:30:15.123Z |
Duration | Represents an amount of time (e.g., “2 hours”) | Duration.ofHours(2) |
Period | Represents a date-based amount of time (e.g., “3 years, 2 months”) | Period.ofYears(3) |
DateTimeFormatter | Formats date and time objects into strings | DateTimeFormatter.ofPattern("yyyy-MM-dd") |
2. Getting the Current Date & Time
Let’s see how we can retrieve the current date and time using Java 8:
📌 Example: Get the Current Date
import java.time.LocalDate;
public class Main {
public static void main(String[] args) {
LocalDate today = LocalDate.now();
System.out.println("Current Date: " + today);
}
}
✅ Output:
Current Date: 2025-02-10
✔ LocalDate.now()
returns the current date without time.
📌 Example: Get the Current Time
import java.time.LocalTime;
public class Main {
public static void main(String[] args) {
LocalTime now = LocalTime.now();
System.out.println("Current Time: " + now);
}
}
✅ Output:
Current Time: 14:35:27.456
✔ LocalTime.now()
returns only the time, including hours, minutes, seconds, and nanoseconds.
📌 Example: Get Both Date & Time (LocalDateTime
)
import java.time.LocalDateTime;
public class Main {
public static void main(String[] args) {
LocalDateTime dateTime = LocalDateTime.now();
System.out.println("Current Date & Time: " + dateTime);
}
}
✅ Output:
Current Date & Time: 2025-02-10T14:35:27.456
✔ LocalDateTime.now()
combines date and time but does not include a time zone.
3. Formatting Dates (DateTimeFormatter
)
By default, Java prints date-time in ISO 8601 format (yyyy-MM-ddTHH:mm:ss
). To customize it, use DateTimeFormatter
.
📌 Example: Custom Date Formatting
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class Main {
public static void main(String[] args) {
LocalDate today = LocalDate.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd-MM-yyyy");
String formattedDate = today.format(formatter);
System.out.println("Formatted Date: " + formattedDate);
}
}
✅ Output:
Formatted Date: 10-02-2025
✔ DateTimeFormatter.ofPattern("dd-MM-yyyy")
customizes the date format.
4. Parsing Strings to Dates
We can convert a string to a date using DateTimeFormatter
.
📌 Example: Convert a String to a LocalDate
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class Main {
public static void main(String[] args) {
String dateString = "15-08-2025";
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd-MM-yyyy");
LocalDate date = LocalDate.parse(dateString, formatter);
System.out.println("Parsed Date: " + date);
}
}
✅ Output:
Parsed Date: 2025-08-15
✔ The string "15-08-2025"
is converted into a LocalDate
object.
5. Adding & Subtracting Time
Method | Description | Example |
---|---|---|
plusDays(n) | Adds n days | date.plusDays(5) |
minusMonths(n) | Subtracts n months | date.minusMonths(2) |
plusHours(n) | Adds n hours | time.plusHours(3) |
📌 Example: Add and Subtract Dates
import java.time.LocalDate;
public class Main {
public static void main(String[] args) {
LocalDate today = LocalDate.now();
LocalDate nextWeek = today.plusDays(7);
LocalDate lastMonth = today.minusMonths(1);
System.out.println("Today: " + today);
System.out.println("Next Week: " + nextWeek);
System.out.println("Last Month: " + lastMonth);
}
}
✅ Output:
Today: 2025-02-10
Next Week: 2025-02-17
Last Month: 2025-01-10
✔ We used plusDays()
and minusMonths()
to modify dates.
6. Working with Time Zones (ZonedDateTime
)
LocalDateTime
does not store time zones, but ZonedDateTime
does.
📌 Example: Get Current Time in a Specific Time Zone
import java.time.ZonedDateTime;
import java.time.ZoneId;
public class Main {
public static void main(String[] args) {
ZonedDateTime tokyoTime = ZonedDateTime.now(ZoneId.of("Asia/Tokyo"));
System.out.println("Current Time in Tokyo: " + tokyoTime);
}
}
✅ Output:
Current Time in Tokyo: 2025-02-10T23:45:30.123+09:00[Asia/Tokyo]
✔ ZoneId.of("Asia/Tokyo")
gives us the current time in Japan.
Lesson Reflection
- Why is
java.time
better thanjava.util.Date
andCalendar
? - How does immutability improve the reliability of date/time objects?
- Can you think of a real-world scenario where
ZonedDateTime
would be useful?
Example: Convert UTC Date-Time to Central Time (CT – America/Chicago)
The ZonedDateTime
class allows us to convert between time zones easily. Central Time (CT) corresponds to the America/Chicago
time zone in Java.
📌 Convert UTC Date-Time to Central Time (CT – America/Chicago
)
import java.time.ZonedDateTime;
import java.time.ZoneId;
import java.time.LocalDateTime;
public class Main {
public static void main(String[] args) {
// Step 1: Create a LocalDateTime (without timezone)
LocalDateTime localDateTime = LocalDateTime.of(2025, 2, 10, 14, 30); // 2:30 PM
// Step 2: Convert LocalDateTime to UTC (Coordinated Universal Time)
ZonedDateTime utcDateTime = localDateTime.atZone(ZoneId.of("UTC"));
// Step 3: Convert UTC to Central Time (CT - America/Chicago)
ZonedDateTime ctDateTime = utcDateTime.withZoneSameInstant(ZoneId.of("America/Chicago"));
// Output results
System.out.println("UTC Time: " + utcDateTime);
System.out.println("Central Time (CT): " + ctDateTime);
}
}
✅ Example Output (Central Time during Standard Time)
UTC Time: 2025-02-10T14:30Z[UTC]
Central Time (CT): 2025-02-10T08:30-06:00[America/Chicago]
✔ 2025-02-10T14:30Z
(UTC) converts to 2025-02-10T08:30-06:00
(CT -6:00).
🔄 Explanation
- Create a
LocalDateTime
→2025-02-10T14:30
(No timezone). - Assign UTC Time Zone →
ZonedDateTime.of(localDateTime, ZoneId.of("UTC"))
. - Convert to CT (America/Chicago) →
withZoneSameInstant(ZoneId.of("America/Chicago"))
. - Print Results → Shows UTC and CT converted time.
⏳ What Happens During Daylight Saving Time (DST)?
Central Time (CT) follows Daylight Saving Time (CDT, UTC-5) from March to November. If the input date falls in this period, the offset changes from -06:00 to -05:00 automatically.
✅ Example Output (During DST – July 10, 2025)
UTC Time: 2025-07-10T14:30Z[UTC]
Central Time (CT): 2025-07-10T09:30-05:00[America/Chicago]
✔ Java automatically adjusts for daylight saving time (DST).
Key Takeaways
✔ ZonedDateTime
allows timezone conversion easily.
✔ Use withZoneSameInstant()
to adjust time zones correctly.
✔ Handles Daylight Saving Time (DST) automatically.
the next Java 8 feature: Optional Class (java.util.Optional
)