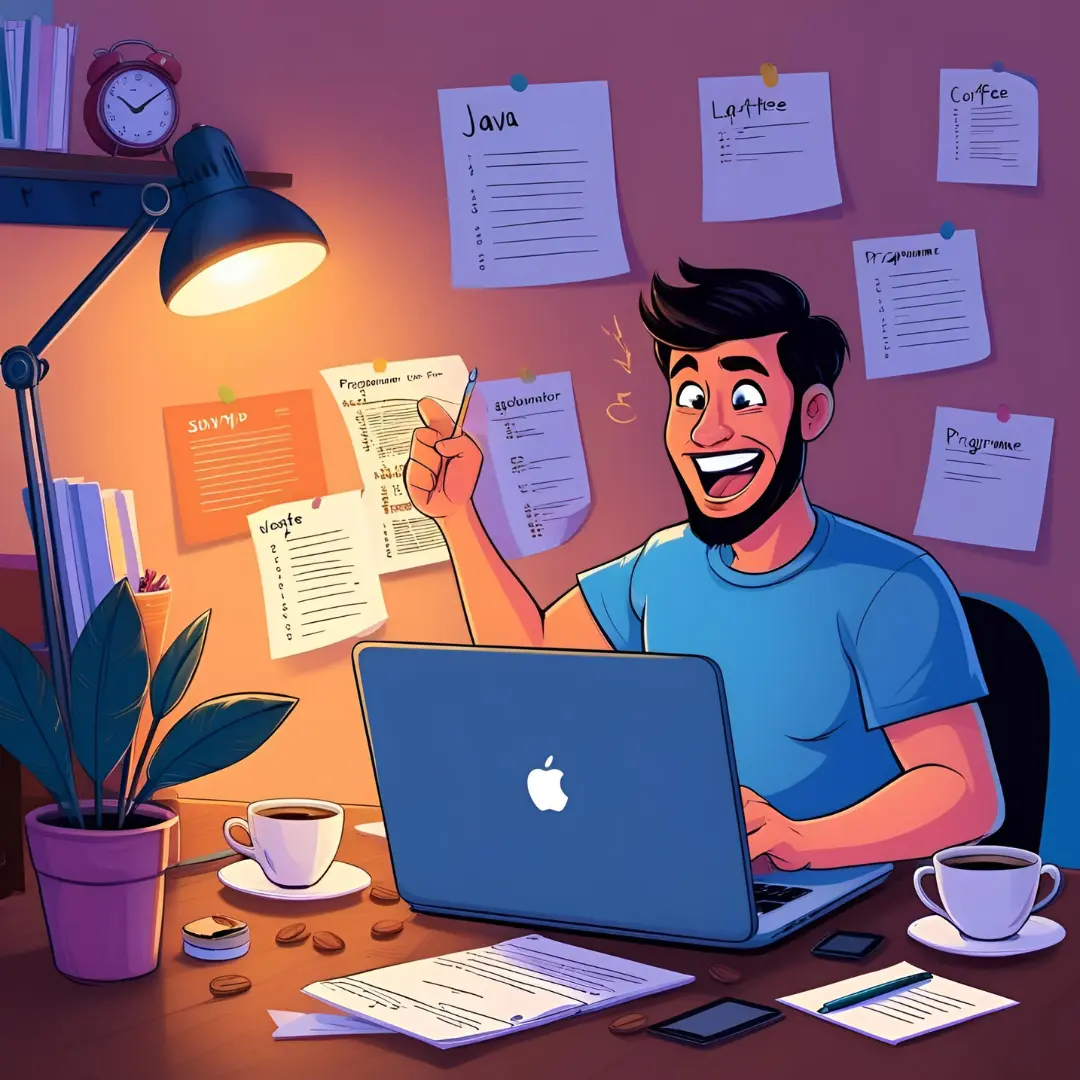
Java 22 expands support for Unnamed Variables (_
) and Unnamed Patterns, building on what Java 21 introduced. This update improves how developers write cleaner, more intention-revealing code—especially in pattern matching and record destructuring.
1. Quick Recap: What Are Unnamed Variables and Patterns?
Unnamed Variable (
_
) – Used when you want to ignore a value Unnamed Pattern (
_
) – Used in pattern matching to ignore a value during destructuring
2. What’s New in Java 22?
Java 22 allows
_
in more places, such as:
- Pattern Matching in
switch
statements instanceof
expressions- Nested record patterns
- Match-all
case _ ->
blocks
3. Example: Using _
in instanceof
Pattern Matching
Before Java 22: Had to name variables, even if unused
if (obj instanceof String s) {
System.out.println("It's a string.");
}
Java 22: Use
_
to ignore the matched value
if (obj instanceof String _) {
System.out.println("It's a string, but I don't need the value.");
}
Clear intent: We care about the type, not the value.
4. Example: switch
with Unnamed Patterns
You can now match types in
switch
, ignore values using _
, and avoid unused variable warnings.
public class Main {
public static void main(String[] args) {
Object input = 42;
switch (input) {
case String s -> System.out.println("String: " + s);
case Integer _ -> System.out.println("An integer (we don't care which one)");
case null -> System.out.println("It's null");
case _ -> System.out.println("Unknown type");
}
}
}
Output:
An integer (we don't care which one)
case _ ->
acts like a default
but is more flexible in pattern matching.
5. Example: Ignoring Nested Record Fields
Records are perfect for pattern matching, and now you can ignore nested fields with
_
.
record Point(int x, int y) {}
record Shape(String type, Point origin) {}
public class Main {
public static void main(String[] args) {
Shape s = new Shape("Circle", new Point(10, 20));
if (s instanceof Shape(String t, Point(_, int y))) {
System.out.println("Shape type: " + t);
System.out.println("Y coordinate: " + y);
}
}
}
Output:
Shape type: Circle
Y coordinate: 20
The
x
coordinate is ignored with _
, keeping the code clean.
6. Benefits of Unnamed Patterns and Variables
Feature | Benefit |
---|---|
case Type _ | Match by type without naming unused values |
instanceof Type _ | Cleaner runtime checks |
case _ | Acts like default , works better with pattern matching |
(_, int y) in records | Only extract what you need, ignore the rest |
7. When Should You Use _
?
Use Unnamed Patterns and Variables when:
- You want simpler, cleaner code in pattern matching
- You only need partial data from a structure (e.g., records)
- You want to avoid unused variable warnings
Don’t use
_
if:
- You need to access the value later in the block
- You’re targeting Java < 21 (not supported)
8. Compilation Note
This feature is still in preview in Java 22.
Use
--enable-preview
when compiling/running:
javac --enable-preview --release 22 Main.java
java --enable-preview Main
Lesson Reflection
- How does using
_
help reduce noise in your code? - When would you use
case _ ->
instead ofdefault
in aswitch
? - Can you think of situations where you’d want to ignore parts of a record or type pattern?
The final round-up/recap of all Java versions and features covered