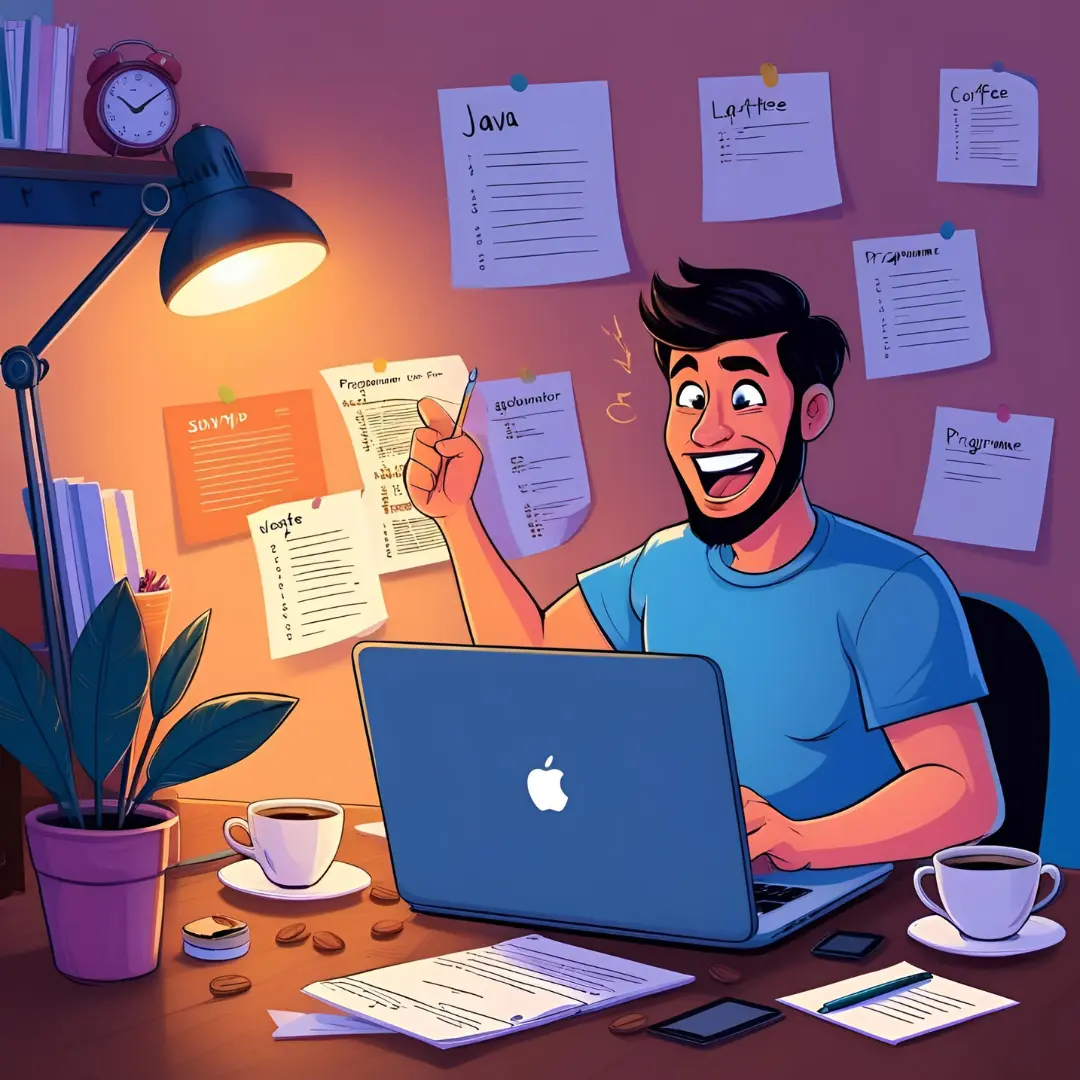
Java 21 introduced Sequenced Collections, a new interface that provides consistent order guarantees for List
, Set
, and Map
. This feature allows more predictable ordering of elements and simplifies common operations like reversing and accessing first/last elements.
1. What Are Sequenced Collections?
✅ A new interface (SequencedCollection<E>
) that extends Collection<E>
.
✅ Ensures a well-defined ordering in List
, Set
, and Map
.
✅ Adds new methods: getFirst()
, getLast()
, reversed()
for easy access.
2. Before Java 21: No Consistent Order in Sets & Maps
🔴 Before Java 21, Set
and Map
had unpredictable iteration order (except Linked variants).
📌 Example: Before Java 21 (Order Not Guaranteed in Set
)
import java.util.Set;
public class Main {
public static void main(String[] args) {
Set<String> set = Set.of("Apple", "Banana", "Cherry");
System.out.println(set); // ❌ Order may change across runs!
}
}
🚨 Output may vary:
[Cherry, Apple, Banana] // First run
[Banana, Cherry, Apple] // Second run
✔ No reliable way to access the first or last element.
3. Java 21+ Solution: Using SequencedCollection
for Ordered Access
🟢 Now, Set
and Map
have predictable order and direct element access!
📌 Example: Accessing First & Last Elements with getFirst()
& getLast()
import java.util.SequencedCollection;
import java.util.List;
public class Main {
public static void main(String[] args) {
SequencedCollection<String> fruits = List.of("Apple", "Banana", "Cherry");
System.out.println("First: " + fruits.getFirst()); // ✅ "Apple"
System.out.println("Last: " + fruits.getLast()); // ✅ "Cherry"
}
}
✅ Output:
First: Apple
Last: Cherry
✔ No need for list.get(0)
or list.get(list.size()-1)
anymore!
4. reversed()
– Easily Reverse Lists, Sets, and Maps
📌 Example: Reversing a List with reversed()
import java.util.SequencedCollection;
import java.util.List;
public class Main {
public static void main(String[] args) {
SequencedCollection<String> fruits = List.of("Apple", "Banana", "Cherry");
System.out.println("Reversed: " + fruits.reversed()); // ✅ ["Cherry", "Banana", "Apple"]
}
}
✅ Output:
Reversed: [Cherry, Banana, Apple]
✔ No need for Collections.reverse()
anymore!
📌 Example: Reversing a Map
Using reversed()
import java.util.SequencedMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
SequencedMap<Integer, String> users = Map.of(1, "Alice", 2, "Bob", 3, "Charlie");
System.out.println("Original: " + users);
System.out.println("Reversed: " + users.reversed()); // ✅ Reverse order!
}
}
✅ Output:
Original: {1=Alice, 2=Bob, 3=Charlie}
Reversed: {3=Charlie, 2=Bob, 1=Alice}
✔ No need for manual sorting—reversing is built-in!
5. When Should You Use Sequenced Collections?
🚀 Use SequencedCollection
when:
✔ You need consistent order in Set
or Map
.
✔ You frequently access the first and last elements.
✔ You want built-in reversal instead of using Collections.reverse()
.
🚨 Do NOT use if:
❌ You are using Java 20 or earlier (Not available).
❌ You don’t need ordered element access.
6. Comparison: Before Java 21 vs. Java 21+ (SequencedCollection
)
Feature | Before Java 21 | Java 21+ (SequencedCollection ) |
---|---|---|
Set/Map Order Guarantee | ❌ No guarantee (except Linked* variants) | ✅ Guaranteed order |
Get First/Last Element | ❌ No direct method | ✅ getFirst() , getLast() |
Reverse Order | ❌ Manual sorting needed | ✅ reversed() built-in |
Lesson Reflection
- How does
SequencedCollection
improve working withSet
andMap
? - Why is
getFirst()
andgetLast()
useful compared tolist.get(0)
? - Can you think of a real-world scenario where reversing a
Map
withreversed()
would be helpful?
Example: Using SequencedCollection
with HashSet
and HashMap
(Java 21+)
Since HashSet
and HashMap
do not guarantee order, they do not implement SequencedCollection
or SequencedMap
. Instead, Java 21+ provides LinkedHashSet
and LinkedHashMap
as SequencedCollection
and SequencedMap
implementations, ensuring predictable iteration order.
📌 Example 1: Using SequencedCollection
with LinkedHashSet
📌 Java 21 allows getFirst()
, getLast()
, and reversed()
on LinkedHashSet
import java.util.SequencedCollection;
import java.util.LinkedHashSet;
public class Main {
public static void main(String[] args) {
SequencedCollection<String> fruits = new LinkedHashSet<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
System.out.println("Original: " + fruits);
System.out.println("First: " + fruits.getFirst()); // ✅ "Apple"
System.out.println("Last: " + fruits.getLast()); // ✅ "Cherry"
System.out.println("Reversed: " + fruits.reversed()); // ✅ ["Cherry", "Banana", "Apple"]
}
}
✅ Output:
Original: [Apple, Banana, Cherry]
First: Apple
Last: Cherry
Reversed: [Cherry, Banana, Apple]
✔ Unlike HashSet
, LinkedHashSet
maintains insertion order and supports SequencedCollection
methods.
📌 Example 2: Using SequencedMap
with LinkedHashMap
📌 Java 21+ allows getFirstEntry()
, getLastEntry()
, and reversed()
on LinkedHashMap
import java.util.SequencedMap;
import java.util.LinkedHashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
SequencedMap<Integer, String> users = new LinkedHashMap<>();
users.put(1, "Alice");
users.put(2, "Bob");
users.put(3, "Charlie");
System.out.println("Original: " + users);
System.out.println("First Entry: " + users.getFirstEntry()); // ✅ {1=Alice}
System.out.println("Last Entry: " + users.getLastEntry()); // ✅ {3=Charlie}
System.out.println("Reversed: " + users.reversed()); // ✅ Reverse order
}
}
✅ Output:
Original: {1=Alice, 2=Bob, 3=Charlie}
First Entry: 1=Alice
Last Entry: 3=Charlie
Reversed: {3=Charlie, 2=Bob, 1=Alice}
✔ Unlike HashMap
, LinkedHashMap
maintains insertion order and supports SequencedMap
methods.
🚨 Why Can’t We Use HashSet
or HashMap
with SequencedCollection
?
🚨 HashSet
and HashMap
do NOT maintain order, so they do not implement SequencedCollection
or SequencedMap
.
🚀 Use LinkedHashSet
or LinkedHashMap
instead for predictable order!
🔍 Key Takeaways
✔ LinkedHashSet
implements SequencedCollection
, allowing getFirst()
, getLast()
, and reversed()
.
✔ LinkedHashMap
implements SequencedMap
, allowing getFirstEntry()
, getLastEntry()
, and reversed()
.
✔ HashSet
and HashMap
do NOT support these operations because they do not maintain order.
The next Java 21+ feature: Structured Concurrency (StructuredTaskScope
) for parallel programming 😊🚀