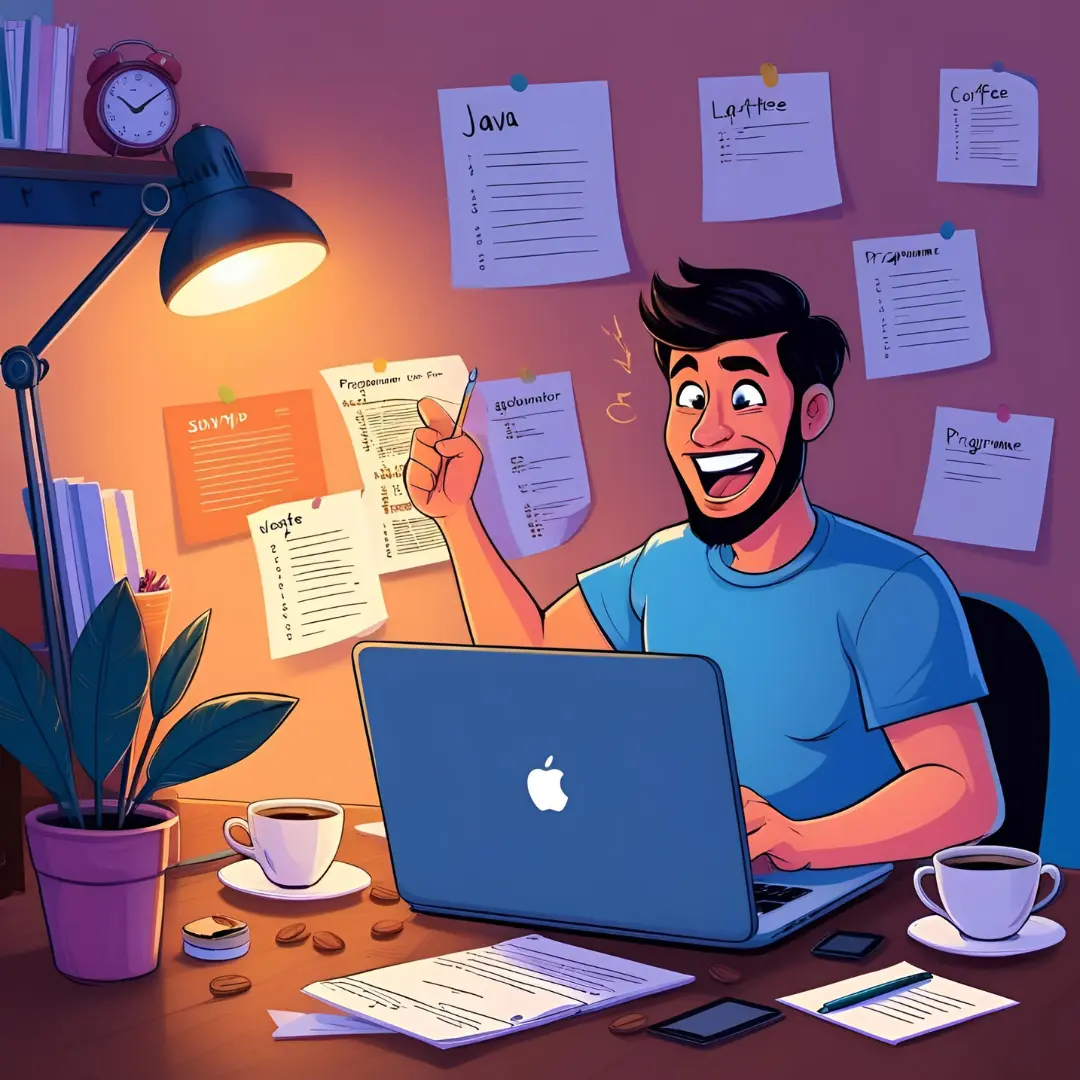
Java 21 introduced String Templates (STR.
), which provide a more readable and safer way to format strings dynamically. Instead of using +
concatenation or String.format()
, STR.
allows embedded expressions inside strings, making them cleaner and more efficient.
1. What Are String Templates (STR.
)?
✅ A new way to embed variables inside a string using STR."""
.
✅ More readable than +
concatenation or String.format()
.
✅ Safer and reduces errors (no need to manually concatenate strings).
✅ Supports inline expressions ({}
) inside the string.
2. Before Java 21: Traditional String Concatenation
🔴 Before Java 21, formatting strings required:
- Manual concatenation (
+
) String.format()
(Less readable, easy to mess up placeholders)
📌 Example: Before Java 21 (Messy String Formatting)
public class Main {
public static void main(String[] args) {
String name = "Alice";
int age = 25;
// ❌ Hard to read, error-prone
String message = "Hello, " + name + "! You are " + age + " years old.";
System.out.println(message);
// ❌ Using String.format (Better, but still complex)
String formattedMessage = String.format("Hello, %s! You are %d years old.", name, age);
System.out.println(formattedMessage);
}
}
✅ Output:
Hello, Alice! You are 25 years old.
Hello, Alice! You are 25 years old.
✔ Works, but is harder to read and maintain.
3. Java 21+ Solution: String Templates (STR.
)
🟢 Now you can embed variables directly in a string!
📌 Example: Using STR.
for Cleaner Formatting
import static java.lang.StringTemplate.STR; // ✅ Import required (Java 21+)
public class Main {
public static void main(String[] args) {
String name = "Alice";
int age = 25;
String message = STR."Hello, \{name}! You are \{age} years old.";
System.out.println(message);
}
}
✅ Output:
Hello, Alice! You are 25 years old.
✔ No +
needed! Expressions are embedded directly into the string.
✔ More readable and safer!
4. Using Expressions Inside STR.
Templates
📌 Example: Inline Calculations in String Templates
public class Main {
public static void main(String[] args) {
int price = 100;
double discount = 0.2;
String message = STR."Final price: \{price - (price * discount)} USD";
System.out.println(message);
}
}
✅ Output:
Final price: 80.0 USD
✔ You can perform calculations directly inside STR.
templates!
5. Combining Multi-line Strings with Templates
🟢 String Templates (STR.
) also work with Text Blocks ("""
)
📌 Example: Using STR.
with Multi-line Text Blocks
public class Main {
public static void main(String[] args) {
String name = "Alice";
int score = 95;
String report = STR."""
Student Report
---------------
Name: \{name}
Score: \{score}
""";
System.out.println(report);
}
}
✅ Output:
Student Report
---------------
Name: Alice
Score: 95
✔ Perfect for generating structured reports and formatted text.
6. When Should You Use String Templates (STR.
)?
🚀 Use STR.
when:
✔ You need clean and readable string formatting.
✔ You want to embed variables directly inside strings.
✔ You work with multi-line text like JSON, SQL, or logs.
🚨 Do NOT use STR.
if:
❌ You are using Java 20 or earlier (Not available).
❌ You need advanced formatting like number padding (Use String.format()
instead).
7. Comparison: Before Java 21 vs. Java 21+ (STR.
)
Feature | Before Java 21 | Java 21+ (STR. ) |
---|---|---|
String Concatenation | + operator (error-prone) | ✅ Inline {} expressions |
String Formatting | String.format("%s %d", var1, var2) | ✅ STR."Hello, {var}!" |
Multi-line Support | Requires \n or manual + | ✅ Works with """ blocks |
Lesson Reflection
- How does
STR.
make string formatting easier compared to concatenation? - Why is
STR.
safer thanString.format()
when handling dynamic text? - Can you think of a scenario where
STR.
would be useful for generating text?
Answers to Reflection Questions on String Templates (STR.
) – Java 21+
1️⃣ How does STR.
make string formatting easier compared to concatenation?
✅ STR.
eliminates the need for +
concatenation, making code cleaner and more readable.
✅ Variables and expressions are directly embedded in the string, reducing errors.
📌 Example: Before vs. After
🔴 Before Java 21 (Messy Concatenation)
String name = "Alice";
int age = 25;
String message = "Hello, " + name + "! You are " + age + " years old.";
System.out.println(message);
🟢 Java 21+ (STR.
is Cleaner!)
String message = STR."Hello, \{name}! You are \{age} years old.";
System.out.println(message);
✅ Output (Same for both cases):
Hello, Alice! You are 25 years old.
✔ No +
operators, fewer mistakes, and easier to read!
2️⃣ Why is STR.
safer than String.format()
when handling dynamic text?
✅ STR.
prevents placeholder mismatches (e.g., forgetting to pass arguments in String.format
).
✅ With String.format()
, argument order errors can occur, leading to incorrect outputs.
📌 Example: Potential Issue with String.format()
String message = String.format("Hello, %s! You are %d years old.", 25, "Alice");
// ❌ ERROR: Wrong argument order, will cause runtime issue!
✅ With STR.
, the variables are inserted directly into the string, avoiding such issues.
String message = STR."Hello, \{name}! You are \{age} years old.";
✔ No argument order mistakes, no missing placeholders!
3️⃣ Can you think of a scenario where STR.
would be useful for generating text?
🚀 Scenario 1: Generating JSON Dynamically
✔ Before Java 21, JSON strings required escape characters and concatenation.
✔ With STR.
, JSON formatting becomes easier.
📌 Example: Creating JSON Strings Dynamically
String name = "Alice";
int age = 25;
String json = STR."""
{
"name": "\{name}",
"age": \{age}
}
""";
System.out.println(json);
✅ Output:
{
"name": "Alice",
"age": 25
}
✔ Much cleaner than manual string concatenation!
🚀 Scenario 2: Generating SQL Queries Dynamically
✔ STR.
makes writing SQL queries easier and less error-prone.
📌 Example: Using STR.
for SQL Queries
String tableName = "users";
String column = "age";
int minAge = 18;
String query = STR."""
SELECT * FROM \{tableName}
WHERE \{column} > \{minAge}
ORDER BY \{column} DESC;
""";
System.out.println(query);
✅ Output:
SELECT * FROM users
WHERE age > 18
ORDER BY age DESC;
✔ No more +
concatenation, no need for manual escaping!
🔍 Key Takeaways
✔ STR.
improves readability and reduces formatting errors.
✔ It is safer than String.format()
, preventing placeholder mismatches.
✔ Perfect for generating structured text like JSON, SQL, and reports.
The next Java 21+ feature: Unnamed Variables & Patterns (_
for unused values) 😊🚀