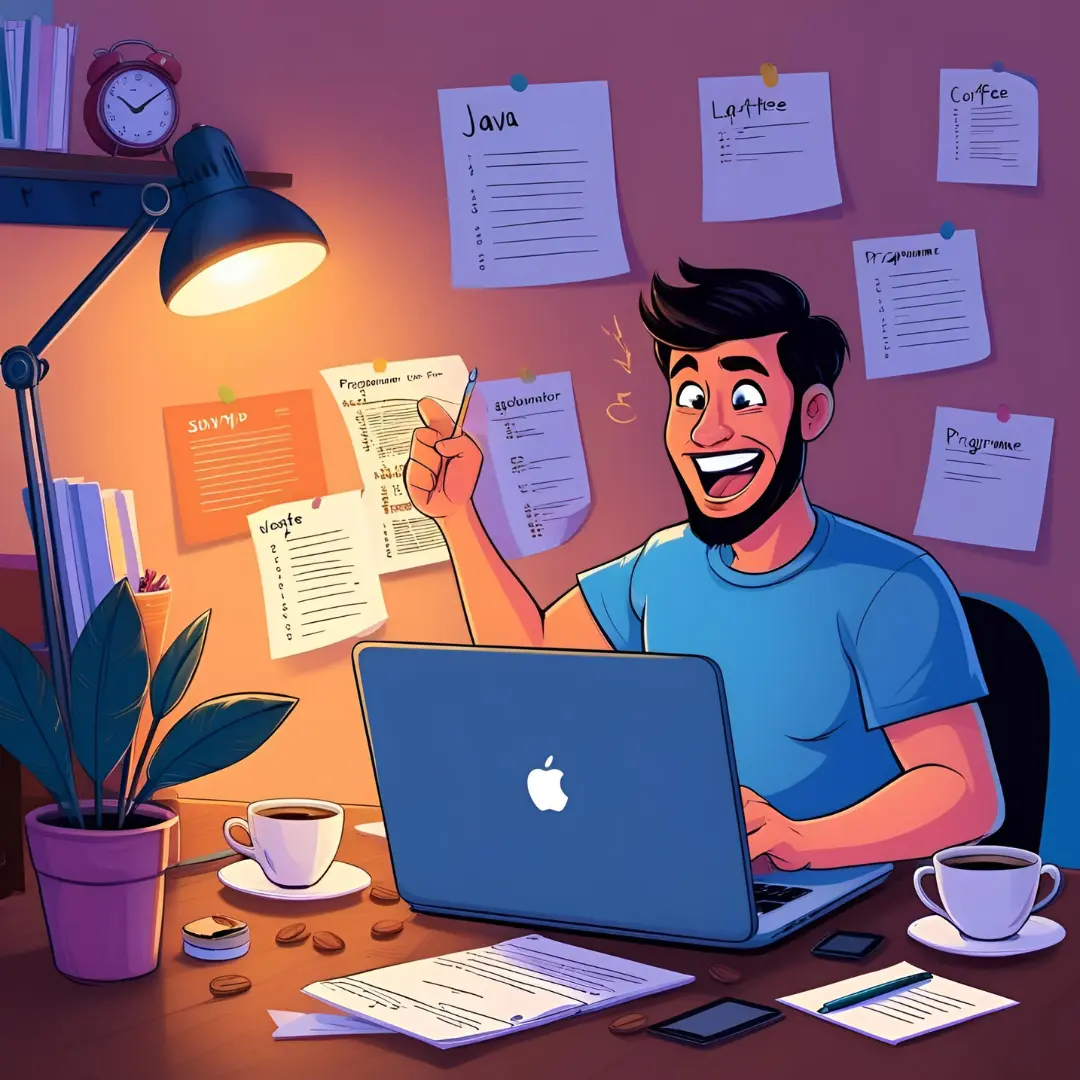
Java 17 introduced Pattern Matching in switch
, allowing switch
statements to handle multiple types and conditions more effectively. This feature eliminates the need for manual type checking and casting, making the code cleaner and safer.
1. What Is Pattern Matching in switch
?
✅ Allows different types in switch
cases (String
, Integer
, Double
, etc.).
✅ Removes the need for instanceof
checks and manual casting.
✅ Supports case null
to handle null
safely.
✅ Supports arrow syntax (->
) for more concise code.
2. Before Java 17: Traditional switch
(Limited to Primitives & Enums)
🔴 Before Java 17, switch
only worked with:
✔ int
, char
, String
, enum
(Objects required instanceof
).
public class Main {
public static void main(String[] args) {
Object obj = "Hello";
if (obj instanceof String) {
String str = (String) obj; // ❌ Manual casting required
System.out.println("String length: " + str.length());
} else if (obj instanceof Integer) {
Integer num = (Integer) obj; // ❌ Manual casting required
System.out.println("Number squared: " + (num * num));
} else {
System.out.println("Unknown type");
}
}
}
✔ Works, but requires explicit instanceof
checks and casting.
3. Java 17+ Solution: Pattern Matching in switch
🟢 Now switch
can check types and cast automatically!
public class Main {
public static void main(String[] args) {
Object obj = "Hello, Java 17!";
switch (obj) {
case String s -> System.out.println("String length: " + s.length());
case Integer i -> System.out.println("Integer squared: " + (i * i));
case Double d -> System.out.println("Double value: " + d);
case null -> System.out.println("It's null!");
default -> System.out.println("Unknown type");
}
}
}
✅ Output (when obj = "Hello, Java 17!"
):
String length: 15
✔ No need for instanceof
or manual casting!
4. case null
– Handling Null Values in switch
🚨 Before Java 17, switch
would throw a NullPointerException
if the value was null
.
📌 Example: Safe Null Handling in switch
(Java 17+)
public class Main {
public static void main(String[] args) {
Object obj = null;
switch (obj) {
case null -> System.out.println("It's null!"); // ✅ Handles null safely
case String s -> System.out.println("String: " + s);
default -> System.out.println("Other type");
}
}
}
✅ Output:
It's null!
✔ No more NullPointerException
!
5. When Should You Use Pattern Matching in switch
?
🚀 Use this feature when:
✔ You need to handle multiple types in a switch
.
✔ You want automatic type casting without instanceof
.
✔ You need to safely handle null
cases.
🚨 Do NOT use if:
❌ You are using Java 16 or earlier (this feature is Java 17+ only).
6. Comparison: Before Java 17 vs. Java 17+ (switch
)
Feature | Before Java 17 | Java 17+ Pattern Matching |
---|---|---|
Supports objects? | ❌ No (switch only worked with String , int , enum ) | ✅ Yes (Works with Object ) |
Automatic casting? | ❌ No (Manual casting required) | ✅ Yes (Automatic casting) |
Handles null ? | ❌ No (NullPointerException if null ) | ✅ Yes (case null supported) |
Lesson Reflection
- How does Pattern Matching in
switch
make code cleaner? - Why does Java 17 allow
null
inswitch
, and how does it improve safety? - Can you think of a real-world use case where Pattern Matching in
switch
would be useful?
The next Java 19+ feature: Virtual Threads (Project Loom
for lightweight concurrency) 😊🚀