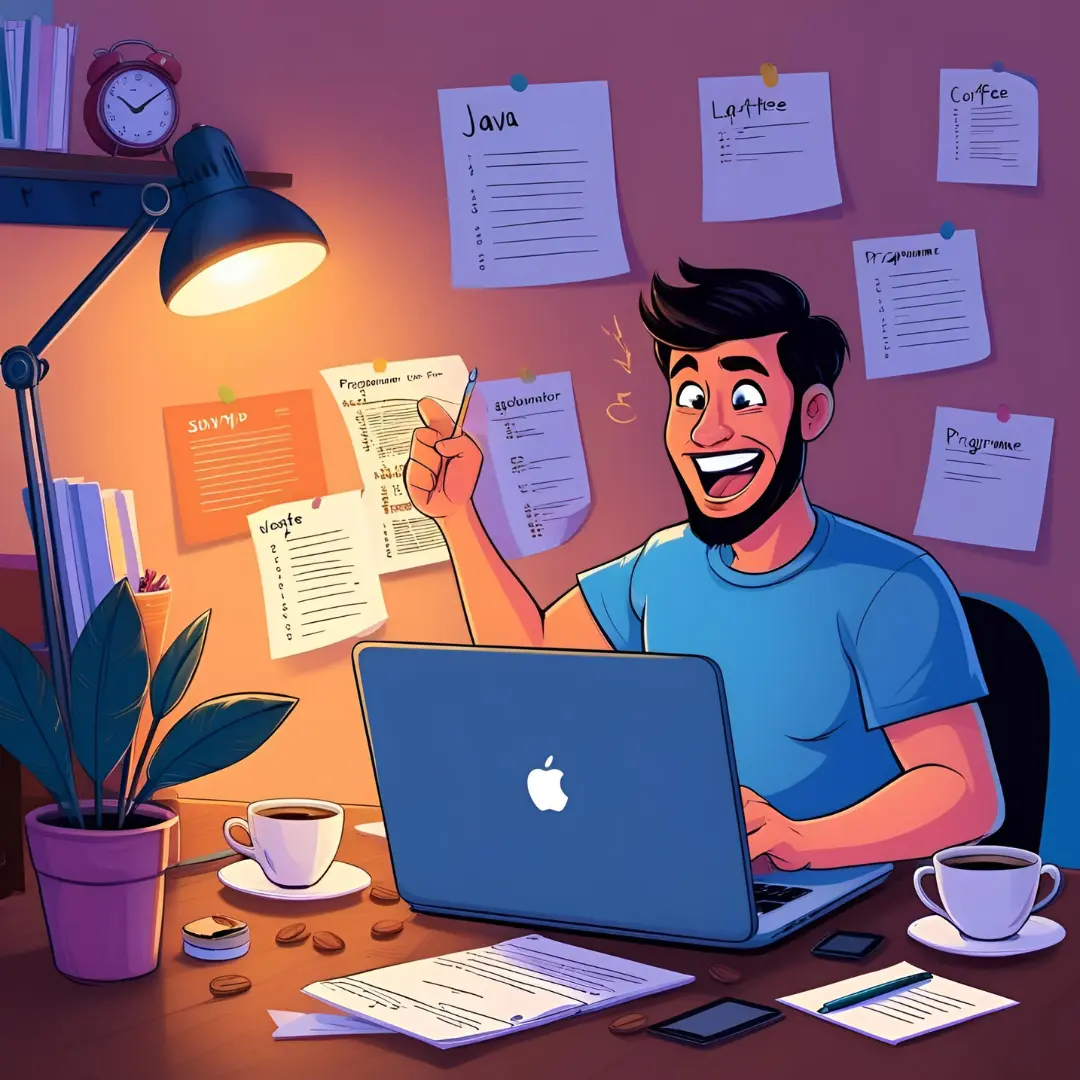
Java 14 introduced Pattern Matching for instanceof
, simplifying type checking and casting. Before Java 14, developers had to manually cast objects after using instanceof
. Now, Java automatically casts the variable, making the code cleaner and safer.
1. What Is Pattern Matching for instanceof
?
✅ Simplifies code by eliminating explicit casting.
✅ Automatically casts the object if instanceof
is true.
✅ Improves readability and reduces redundant code.
2. Before Java 14 (Manual Casting Required)
🔴 Older way (Java 13 and below)
public class Main {
public static void main(String[] args) {
Object obj = "Hello, Java 14!";
if (obj instanceof String) { // ✅ Check type
String str = (String) obj; // ❌ Manual cast required
System.out.println(str.toUpperCase());
}
}
}
✅ Output:
HELLO, JAVA 14!
✔ Explicit (String) obj
cast is required.
3. Java 14+ (Pattern Matching Simplifies instanceof
)
🟢 New way (Java 14+ with Pattern Matching)
public class Main {
public static void main(String[] args) {
Object obj = "Hello, Java 14!";
if (obj instanceof String str) { // ✅ Auto-cast with variable declaration
System.out.println(str.toUpperCase()); // No need to cast manually!
}
}
}
✅ Output:
HELLO, JAVA 14!
✔ No explicit cast needed! str
is automatically a String
.
4. Benefits of Pattern Matching for instanceof
Feature | Before Java 14 | **Java 14+ (instanceof Pattern Matching) |
---|---|---|
Explicit Casting | ✅ Required | ❌ Not needed |
Code Readability | ❌ More verbose | ✅ Cleaner and more readable |
Variable Scope | ❌ New variable needed | ✅ Defined directly in instanceof |
Null Safety | ❌ Can cause NullPointerException | ✅ Safer (null is checked automatically) |
5. Scope of Pattern Matching Variables
✅ The variable declared inside instanceof
is only accessible within the if
block.
🔴 Example: Pattern Variable Out of Scope
public class Main {
public static void main(String[] args) {
Object obj = "Java 14 Pattern Matching";
if (obj instanceof String str) {
System.out.println(str.toUpperCase());
}
// System.out.println(str); // ❌ ERROR: str is not accessible here!
}
}
🚨 Error: 'str' cannot be resolved outside the if block.
✔ Pattern variables exist only inside the if
block where they are defined.
6. Pattern Matching Works with else
and switch
📌 Example: Using Pattern Matching with else
public class Main {
public static void main(String[] args) {
Object obj = 42; // This is an Integer
if (obj instanceof String str) {
System.out.println("String length: " + str.length());
} else if (obj instanceof Integer num) {
System.out.println("Double value: " + (num * 2));
}
}
}
✅ Output:
Double value: 84
✔ No need for explicit casting!
📌 Example: Pattern Matching with switch
(Java 17+)
Pattern Matching is also available in Java 17+ switch
statements:
public class Main {
public static void main(String[] args) {
Object obj = 3.14;
switch (obj) {
case String s -> System.out.println("String: " + s.toUpperCase());
case Integer i -> System.out.println("Integer squared: " + (i * i));
case Double d -> System.out.println("Double value: " + d);
default -> System.out.println("Unknown type");
}
}
}
✅ Output:
Double value: 3.14
✔ Pattern Matching in switch
removes the need for instanceof
checks manually!
Lesson Reflection
- How does Pattern Matching for
instanceof
make code more readable? - Why is explicit casting no longer required in Java 14+?
- What happens if you try to use the pattern variable (
str
) outside theif
block?
The next Java 15+ feature: Sealed Classes (sealed
, permits
)? 😊🚀