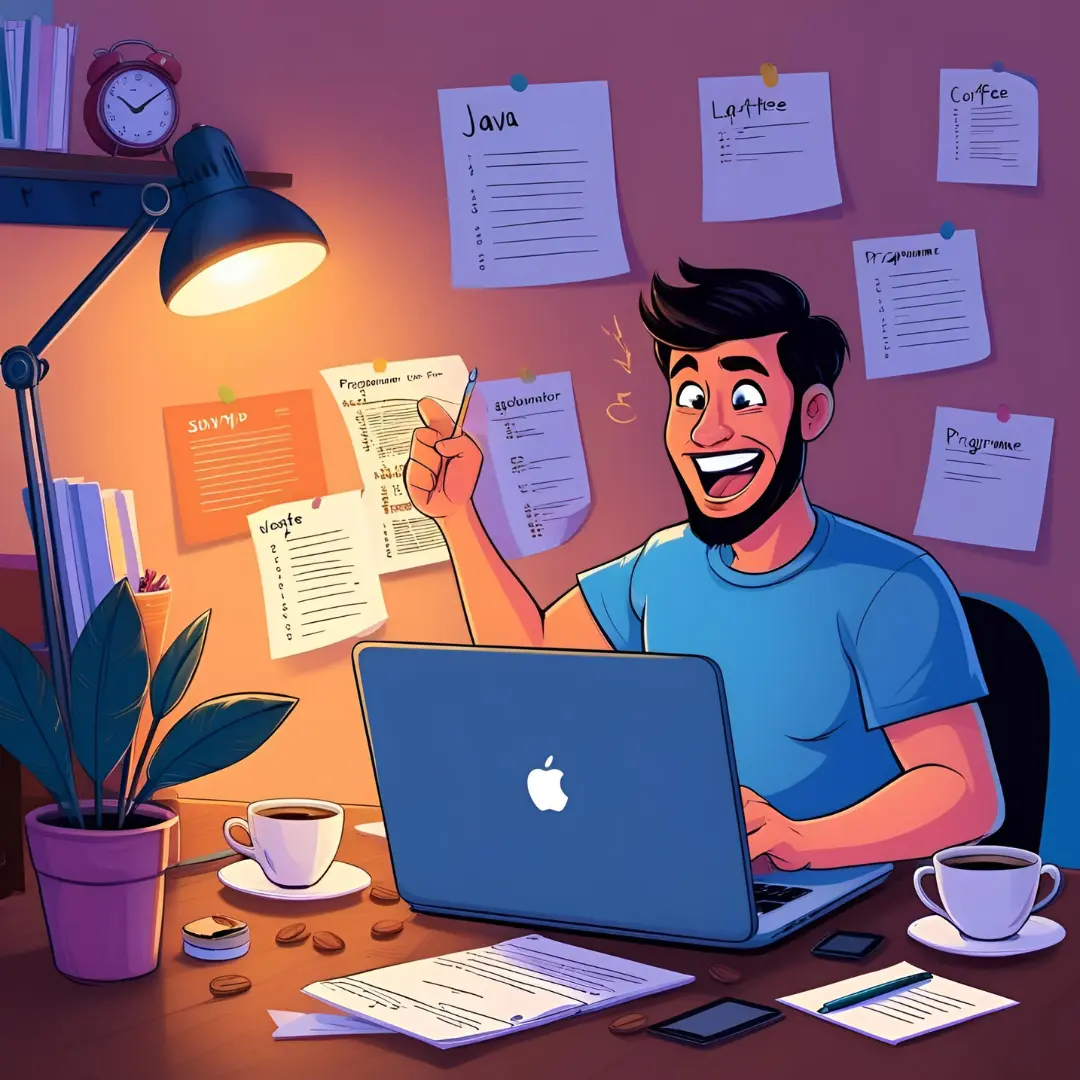
Java 13 introduced Text Blocks, a new way to write multi-line strings using triple quotes ("""
). This feature makes it easier to write formatted text such as JSON, XML, HTML, and SQL queries.
1. What Are Text Blocks?
✅ Text Blocks allow multi-line strings without using \n
.
✅ They preserve line breaks and indentation for better readability.
✅ They remove the need for manual string concatenation (+
).
2. How to Use Text Blocks ("""
)?
📌 Example: Defining a Text Block
public class Main {
public static void main(String[] args) {
String text = """
This is a text block.
It spans multiple lines.
No need for \\n or +.
""";
System.out.println(text);
}
}
✅ Output:
This is a text block.
It spans multiple lines.
No need for \n or +.
✔ Preserves line breaks automatically!
3. Comparison: Before Java 13 vs. Java 13+ Text Blocks
🔴 Before Java 13 (Verbose & Hard to Read)
String json = "{\n" +
" \"name\": \"Alice\",\n" +
" \"age\": 25\n" +
"}";
🟢 Java 13+ (Cleaner & Readable)
String json = """
{
"name": "Alice",
"age": 25
}
""";
✔ No need for \n
or +
!
4. Text Blocks for SQL Queries
📌 Example: SQL Query Before vs. After
🔴 Before Java 13
String query = "SELECT * FROM users " +
"WHERE age > 18 " +
"ORDER BY name;";
🟢 With Java 13+ Text Blocks
String query = """
SELECT * FROM users
WHERE age > 18
ORDER BY name;
""";
✔ Easier to read and maintain!
5. Formatting and Indentation in Text Blocks
✅ Text Blocks automatically remove common leading spaces.
📌 Example: Automatic Indentation Handling
public class Main {
public static void main(String[] args) {
String html = """
<html>
<body>
<h1>Hello, Java 13!</h1>
</body>
</html>
""";
System.out.println(html);
}
}
✅ Output:
<html>
<body>
<h1>Hello, Java 13!</h1>
</body>
</html>
✔ Maintains proper indentation without extra spaces.
6. Escape Sequences in Text Blocks
🚀 Most escape sequences (\t
, \n
, \"
, etc.) work normally.
📌 Example: Using Escape Characters in Text Blocks
String text = """
This is a "quoted" text block.
This line has a tab:\tIndented!
""";
System.out.println(text);
✅ Output:
This is a "quoted" text block.
This line has a tab: Indented!
✔ Text Blocks work with escape sequences just like normal strings!
Lesson Reflection
- How do Text Blocks improve the readability of multi-line strings?
- When would you use a Text Block instead of a regular string?
- How does Java handle indentation inside a Text Block?
Answers to Reflection Questions on Text Blocks ("""
)
1️⃣ How do Text Blocks improve the readability of multi-line strings?
✅ Before Java 13, multi-line strings required \n
and +
, making them hard to read.
✅ Text Blocks ("""
) allow natural multi-line formatting without extra characters.
📌 Example: JSON String Before vs. After
🔴 Before Java 13 (Hard to Read)
String json = "{\n" +
" \"name\": \"Alice\",\n" +
" \"age\": 25\n" +
"}";
🟢 With Java 13+ (Text Block is Cleaner)
String json = """
{
"name": "Alice",
"age": 25
}
""";
✔ No need for \n
or +
, and it looks like real JSON!
2️⃣ When would you use a Text Block instead of a regular string?
🚀 Use a Text Block when dealing with:
✅ Multi-line text content – JSON, XML, HTML, SQL, logs, emails.
✅ Formatted strings that need indentation – Helps maintain structure.
✅ Large blocks of text – Avoids cluttered concatenation.
📌 Example: Using Text Blocks for SQL Queries
🔴 Before Java 13 (Messy Concatenation)
String query = "SELECT * FROM users " +
"WHERE age > 18 " +
"ORDER BY name;";
🟢 With Java 13+ (Much Cleaner!)
String query = """
SELECT * FROM users
WHERE age > 18
ORDER BY name;
""";
✔ Easier to read, write, and maintain!
3️⃣ How does Java handle indentation inside a Text Block?
✅ Java automatically removes common leading spaces to align with the leftmost non-space character.
✅ Indentation inside the text block is preserved.
📌 Example: Automatic Indentation Handling
String html = """
<html>
<body>
<h1>Hello, Java 13!</h1>
</body>
</html>
""";
System.out.println(html);
✅ Output:
<html>
<body>
<h1>Hello, Java 13!</h1>
</body>
</html>
✔ No extra spaces! Java adjusts indentation properly.
🔍 Key Takeaways
✔ Text Blocks ("""
) make multi-line strings more readable and maintainable.
✔ They are perfect for JSON, SQL, HTML, logs, and other formatted text.
✔ Java automatically removes unnecessary leading spaces while preserving indentation.
The next Java 14+ feature: Pattern Matching for instanceof
? 😊🚀