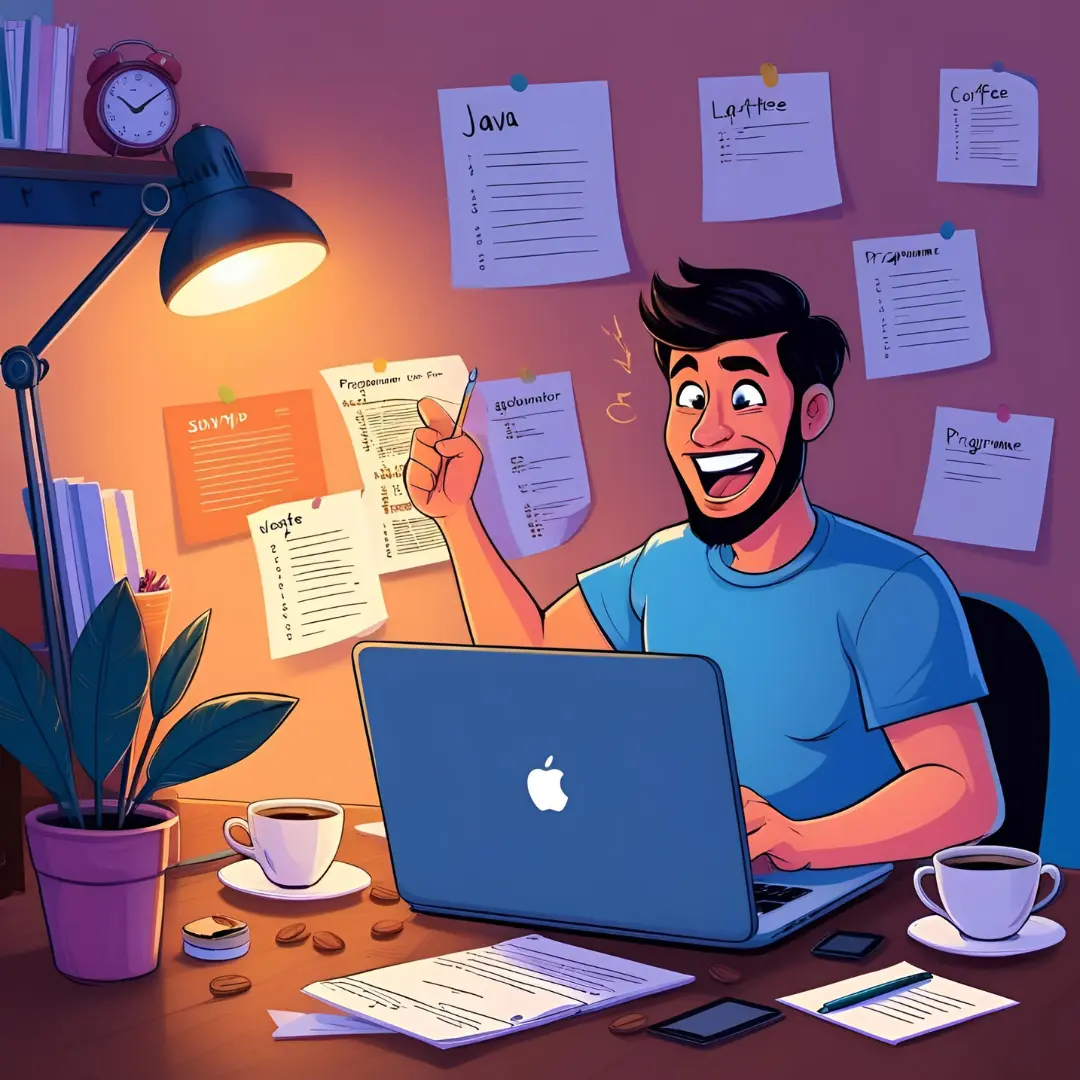
Java 11 introduced simpler ways to read and write files using the Files
class. These new methods improve file handling by reducing boilerplate code.
1. What Are the New File Methods?
Method | Purpose | Example |
---|---|---|
Files.readString(Path) | Reads an entire file into a String | Files.readString(path) |
Files.writeString(Path, String) | Writes a String to a file | Files.writeString(path, "Hello, Java 11!") |
β Why use these methods?
- Before Java 11, reading/writing files required
BufferedReader
orBufferedWriter
. - Now,
readString()
andwriteString()
make it simpler and more readable.
2. Reading a File Using readString()
π Example: Reading a File in Java 11+
import java.nio.file.Files;
import java.nio.file.Path;
public class Main {
public static void main(String[] args) throws Exception {
Path filePath = Path.of("example.txt");
String content = Files.readString(filePath);
System.out.println("File Content:\n" + content);
}
}
β
Output (If example.txt
contains “Hello, World!”)
File Content:
Hello, World!
β Much cleaner than Java 8’s BufferedReader
!
3. Writing to a File Using writeString()
π Example: Writing Text to a File
import java.nio.file.Files;
import java.nio.file.Path;
public class Main {
public static void main(String[] args) throws Exception {
Path filePath = Path.of("example.txt");
Files.writeString(filePath, "Hello, Java 11!");
System.out.println("File written successfully.");
}
}
β Output:
File written successfully.
β No need for FileWriter
or BufferedWriter
!
4. Appending to a File
π Example: Appending Text Using StandardOpenOption.APPEND
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.StandardOpenOption;
public class Main {
public static void main(String[] args) throws Exception {
Path filePath = Path.of("example.txt");
Files.writeString(filePath, "\nAppending new text!", StandardOpenOption.APPEND);
System.out.println("Text appended successfully.");
}
}
β
Output (example.txt
after running the program):
Hello, Java 11!
Appending new text!
β Allows adding new content without overwriting the existing file.
5. Comparison: Java 8 vs. Java 11 File Handling
Feature | Java 8 (Verbose) | Java 11+ (Simplified) |
---|---|---|
Read File | Files.readAllBytes(path) β new String(byte[]) | Files.readString(path) |
Write File | Files.write(path, text.getBytes()) | Files.writeString(path, text) |
Append to File | Files.write(path, text.getBytes(), StandardOpenOption.APPEND) | Files.writeString(path, text, StandardOpenOption.APPEND) |
6. Handling Exceptions with Files.readString()
and Files.writeString()
π¨ What if the file doesnβt exist?
readString()
will throw aNoSuchFileException
.writeString()
will create the file automatically if it doesn’t exist.
π Example: Handling Missing File
import java.nio.file.Files;
import java.nio.file.Path;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
Path filePath = Path.of("missing.txt");
try {
String content = Files.readString(filePath); // β FileNotFound if missing
System.out.println(content);
} catch (IOException e) {
System.out.println("File not found: " + e.getMessage());
}
}
}
β
Output (if missing.txt
does not exist):
File not found: missing.txt
β Use try-catch to handle missing files safely.
Lesson Reflection
- Why are
readString()
andwriteString()
better than older file handling methods? - What happens if you try to read a file that doesnβt exist using
readString()
? - How can you append data to an existing file instead of overwriting it?
The next Java 12+ feature: New Collectors Methods (teeing()
) ππ