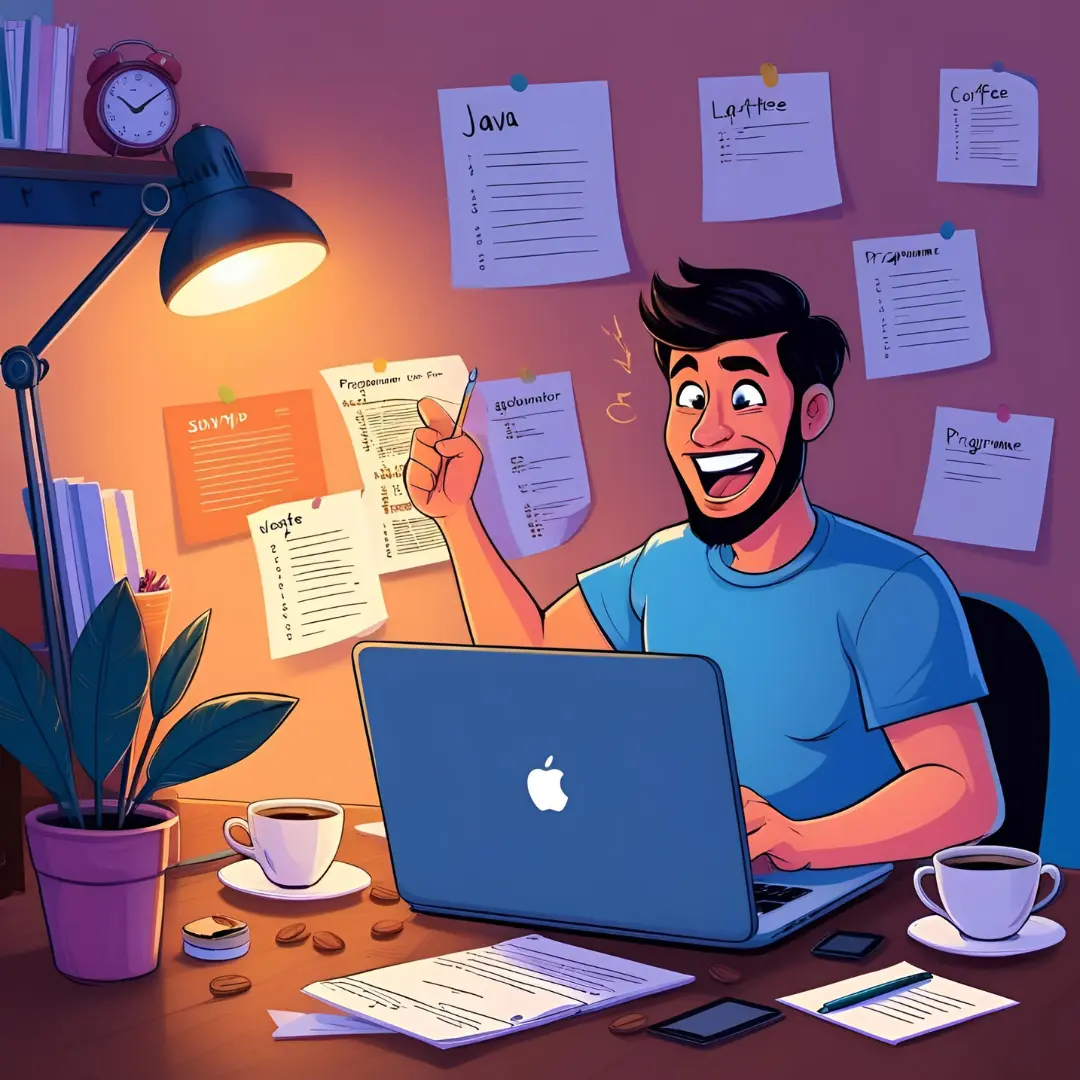
Java 11 introduced new methods for the String
class, making it easier to handle whitespace, multi-line text, and string repetition.
1. strip()
, stripLeading()
, stripTrailing()
(Better Whitespace Handling)
πΉ Before Java 11, trim()
was used to remove spaces, but it only removed ASCII spaces (U+0020
), not Unicode spaces.
πΉ Java 11 introduced strip()
, which removes all leading and trailing Unicode whitespace.
Method | Description | Example |
---|---|---|
strip() | Removes leading and trailing whitespace (Unicode-aware) | " Hello ".strip() β "Hello" |
stripLeading() | Removes leading whitespace only | " Hello ".stripLeading() β "Hello " |
stripTrailing() | Removes trailing whitespace only | " Hello ".stripTrailing() β " Hello" |
π Example 1: Difference Between trim()
and strip()
public class Main {
public static void main(String[] args) {
String s1 = " Hello ";
String s2 = "\u2003Hello\u2003"; // Unicode whitespace
System.out.println("Trim: [" + s2.trim() + "]"); // Doesn't remove Unicode spaces
System.out.println("Strip: [" + s2.strip() + "]"); // β
Removes Unicode spaces
}
}
β Output:
Trim: [βHelloβ]
Strip: [Hello]
β strip()
removes Unicode whitespace, unlike trim()
.
π Example 2: stripLeading()
and stripTrailing()
public class Main {
public static void main(String[] args) {
String text = " Java 11 ";
System.out.println("Strip Leading: [" + text.stripLeading() + "]");
System.out.println("Strip Trailing: [" + text.stripTrailing() + "]");
}
}
β Output:
Strip Leading: [Java 11 ]
Strip Trailing: [ Java 11]
β More control over whitespace removal!
2. lines()
β Splitting Multi-line Strings into a Stream
πΉ Before Java 11, splitting multi-line text required manually handling \n
.
πΉ lines()
simplifies this by converting a multi-line string into a Stream<String>
.
π Example: Using lines()
to Process Multi-Line Text
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
String text = "Java 11\nNew Features\nImproved Performance";
text.lines()
.map(String::toUpperCase) // Convert each line to uppercase
.forEach(System.out::println);
}
}
β Output:
JAVA 11
NEW FEATURES
IMPROVED PERFORMANCE
β lines()
splits the string correctly without manually handling \n
.
3. repeat(int n)
β Repeat Strings Easily
πΉ Before Java 11, repeating a string required loops or StringBuilder
.
πΉ repeat(n)
simplifies this by repeating a string n
times.
π Example: Using repeat()
public class Main {
public static void main(String[] args) {
String star = "*";
System.out.println(star.repeat(5)); // Prints *****
}
}
β Output:
*****
β Simpler than using loops!
π Summary of Java 11+ String Methods
Method | Purpose | Example |
---|---|---|
strip() | Removes leading & trailing Unicode spaces | " Hello ".strip() β "Hello" |
stripLeading() | Removes only leading spaces | " Hello ".stripLeading() β "Hello " |
stripTrailing() | Removes only trailing spaces | " Hello ".stripTrailing() β " Hello" |
lines() | Splits multi-line text into a stream | "A\nB".lines() β Stream(["A", "B"] ) |
repeat(n) | Repeats the string n times | "*".repeat(5) β "*****" |
Lesson Reflection
- Why is
strip()
better thantrim()
for removing whitespace? - How does
lines()
make multi-line text processing easier? - Can you think of a scenario where
repeat()
would be useful?
Answers to Reflection Questions on Java 11+ String Methods
1οΈβ£ Why is strip()
better than trim()
for removing whitespace?
β
strip()
handles Unicode whitespace, while trim()
does not.
trim()
only removes ASCII space (U+0020
).strip()
removes all Unicode whitespace characters (like\u2003
β an invisible space).
π Example: Difference Between trim()
and strip()
public class Main {
public static void main(String[] args) {
String unicodeSpace = "\u2003Hello\u2003"; // Unicode whitespace around "Hello"
System.out.println("Trim: [" + unicodeSpace.trim() + "]"); // β Doesn't remove Unicode spaces
System.out.println("Strip: [" + unicodeSpace.strip() + "]"); // β
Removes Unicode spaces
}
}
β Output:
Trim: [βHelloβ] // β Unicode spaces remain
Strip: [Hello] // β
Unicode spaces removed
β strip()
is more powerful than trim()
because it handles ALL whitespace characters.
2οΈβ£ How does lines()
make multi-line text processing easier?
β
lines()
splits multi-line strings into a Stream<String>
, allowing functional processing.
- Before Java 11, we had to manually split by
\n
and handle edge cases. - Now,
lines()
does the work automatically and ignores empty trailing lines.
π Example: Processing Multi-Line Text with lines()
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
String text = "Java 11\nNew Features\nBetter Performance";
text.lines()
.map(String::toUpperCase) // Convert each line to uppercase
.forEach(System.out::println);
}
}
β Output:
JAVA 11
NEW FEATURES
BETTER PERFORMANCE
β No need to manually split and loop over lines!
3οΈβ£ Can you think of a scenario where repeat()
would be useful?
π Yes! repeat(n)
is useful when generating formatted output.
β Use Case 1: Creating a Separator Line for Console Output
public class Main {
public static void main(String[] args) {
System.out.println("-".repeat(50)); // Prints a 50-character separator line
}
}
β Output:
--------------------------------------------------
β This avoids loops or StringBuilder
just to repeat characters.
β Use Case 2: Generating Test Data
public class Main {
public static void main(String[] args) {
var sampleData = "ABC".repeat(5); // Repeats "ABC" 5 times
System.out.println(sampleData);
}
}
β Output:
ABCABCABCABCABC
β Great for quickly generating repeated patterns in testing.
π Key Takeaways
β strip()
is better than trim()
because it removes all Unicode whitespace.
β lines()
simplifies multi-line text processing using Stream<String>
.
β repeat(n)
eliminates the need for loops when generating repeated strings.
The next Java 11+ feature: New File Methods (readString()
, writeString()
)? ππ