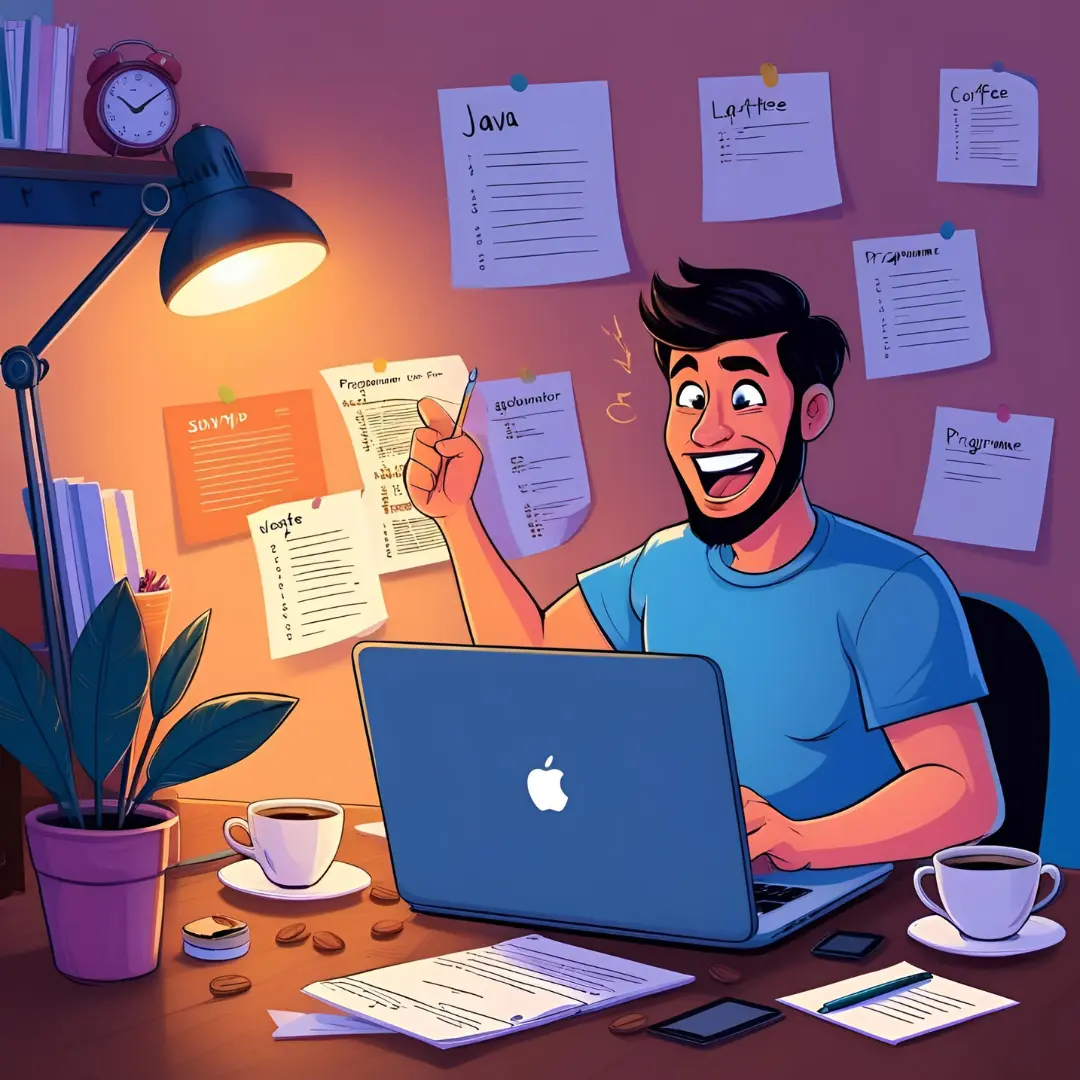
Java 10 introduced local variable type inference using the var
keyword. This feature allows Java to infer the type of a local variable automatically, making the code more concise and readable.
1. What is var
in Java?
✅ Introduced in Java 10, var
allows you to declare local variables without explicitly specifying their type.
✅ Java infers the type from the assigned value.
✅ It does NOT mean Java is dynamically typed! – Java remains statically typed; types are determined at compile-time.
📌 Example: Using var
to Declare Local Variables
public class Main {
public static void main(String[] args) {
var name = "Alice"; // Inferred as String
var age = 25; // Inferred as int
var pi = 3.14; // Inferred as double
System.out.println(name + " is " + age + " years old. Pi = " + pi);
}
}
✅ Output:
Alice is 25 years old. Pi = 3.14
✔ Java automatically infers String
, int
, and double
.
2. Rules & Limitations of var
✅ Allowed:
var
must be initialized at the time of declaration.- It can be used for local variables, loops, and try-with-resources.
❌ Not Allowed:
var
CANNOT be used for method parameters or return types.- It CANNOT be used with
null
alone (type inference fails). - Cannot be used for instance or class variables.
📌 ✅ Allowed: Using var
in a Loop
for (var i = 0; i < 5; i++) {
System.out.println(i);
}
📌 ❌ Not Allowed: Using var
as a Class Field
class Person {
var name; // ❌ ERROR: 'var' is not allowed for class fields
}
✔ var
is only for local variables inside methods!
3. Benefits of Using var
✅ Reduces Code Clutter
🔴 Without var
(Verbose):
Map<String, List<Integer>> map = new HashMap<String, List<Integer>>();
🟢 With var
(Cleaner):
var map = new HashMap<String, List<Integer>>();
✔ Java infers the type automatically, making code shorter and easier to read.
✅ Makes Code More Readable for Long Type Names
📌 Example: Long Generic Type Declaration
🔴 Without var
:
Map<String, List<String>> phoneBook = new HashMap<String, List<String>>();
🟢 With var
:
var phoneBook = new HashMap<String, List<String>>();
✔ Less repetition, better readability!
4. When NOT to Use var
🚨 Avoid var
when it reduces code clarity.
📌 Example: Hard-to-Read Code with var
var x = getValue(); // ❌ What type is x?
✅ Better:
String value = getValue(); // ✅ Explicit type is clearer
✔ Use var
when it improves readability, but avoid it when type clarity is lost.
5. How Does Java Infer Types?
📌 Example: Checking Inferred Types
var a = "Hello"; // Inferred as String
var b = 10; // Inferred as int
var c = 5.5; // Inferred as double
var d = new ArrayList<String>(); // Inferred as ArrayList<String>
✅ Java determines types at compile-time, NOT at runtime!
6. Comparing var
vs. Explicit Typing
Feature | Using var | Explicit Typing |
---|---|---|
Readability | ✅ Cleaner for long type names | ❌ Can be verbose |
Clarity | ❌ Sometimes unclear | ✅ Always clear |
Compile-Time Type Safety | ✅ Fully Type-Safe | ✅ Fully Type-Safe |
Usage in Methods | ❌ Cannot be used for return types | ✅ Can be used everywhere |
✔ Use var
for long type names but prefer explicit types when clarity is needed.
Lesson Reflection
- How does
var
improve code readability compared to explicit types? - Why is
var
not allowed for class fields and method return types? - Can you think of a situation where using
var
might make code harder to understand?
Answers to Reflection Questions on var
1️⃣ How does var
improve code readability compared to explicit types?
✅ Reduces Code Clutter
var
removes unnecessary repetition, making code cleaner and easier to read.- It is especially useful for long generic type declarations.
📌 Example: Without var
(Verbose)
Map<String, List<Integer>> map = new HashMap<String, List<Integer>>();
📌 With var
(Cleaner)
var map = new HashMap<String, List<Integer>>();
✔ Code is shorter and more readable without losing type safety.
✅ Best Used When the Type is Obvious
var name = "Alice"; // Clearly a String
var age = 30; // Clearly an int
var list = List.of(1, 2, 3); // Clearly a List<Integer>
✔ No need to explicitly mention the type since it’s obvious from the assigned value.
2️⃣ Why is var
not allowed for class fields and method return types?
🚨 Reason 1: Java Needs Strong Type Information for Fields and Methods
- Java infers
var
types only inside methods, but fields/methods must have explicit types to ensure clarity. - Since
var
does not specify a type explicitly, allowing it for fields would make the class harder to understand.
📌 Example: Why var
is NOT Allowed for Fields
class Person {
var name; // ❌ ERROR: Type is unknown, making the class unclear
}
✅ Solution (Explicit Type Required for Fields)
Editclass Person {
String name; // ✅ Explicit type ensures clarity
}
✔ Fields must have explicit types to avoid confusion when creating objects.
🚨 Reason 2: Method Return Types Must Be Explicit for API Clarity
- When using a method, the return type should be clearly defined so developers know what to expect.
- Allowing
var
would make method signatures ambiguous.
📌 Example: Why var
is NOT Allowed in Method Return Types
var getName() { // ❌ ERROR: Return type must be specified
return "Alice";
}
✅ Solution (Explicit Return Type Required)
String getName() { // ✅ Explicit return type makes it clear
return "Alice";
}
✔ Explicit return types make APIs easier to use and understand.
3️⃣ Can you think of a situation where using var
might make code harder to understand?
🚨 Yes, when the inferred type is unclear!
📌 Example: var
Makes Type Unclear
var x = getValue(); // ❌ What type is x?
- If
getValue()
returns anint
,String
, orList<>
, it’s not obvious from the code. - This makes debugging and reading the code more difficult.
✅ Solution: Use an Explicit Type When Needed
String value = getValue(); // ✅ Now it's clear that value is a String
✔ Use var
only when it improves readability, not when it creates confusion.
🔍 Key Takeaways
✔ var
improves readability by reducing type clutter, especially for long generic types.
✔ It is not allowed for class fields and method return types to maintain code clarity.
✔ Avoid var
when the inferred type is not obvious.
The next Java 11+ feature: New String Methods (strip()
, lines()
, repeat()
) 😊