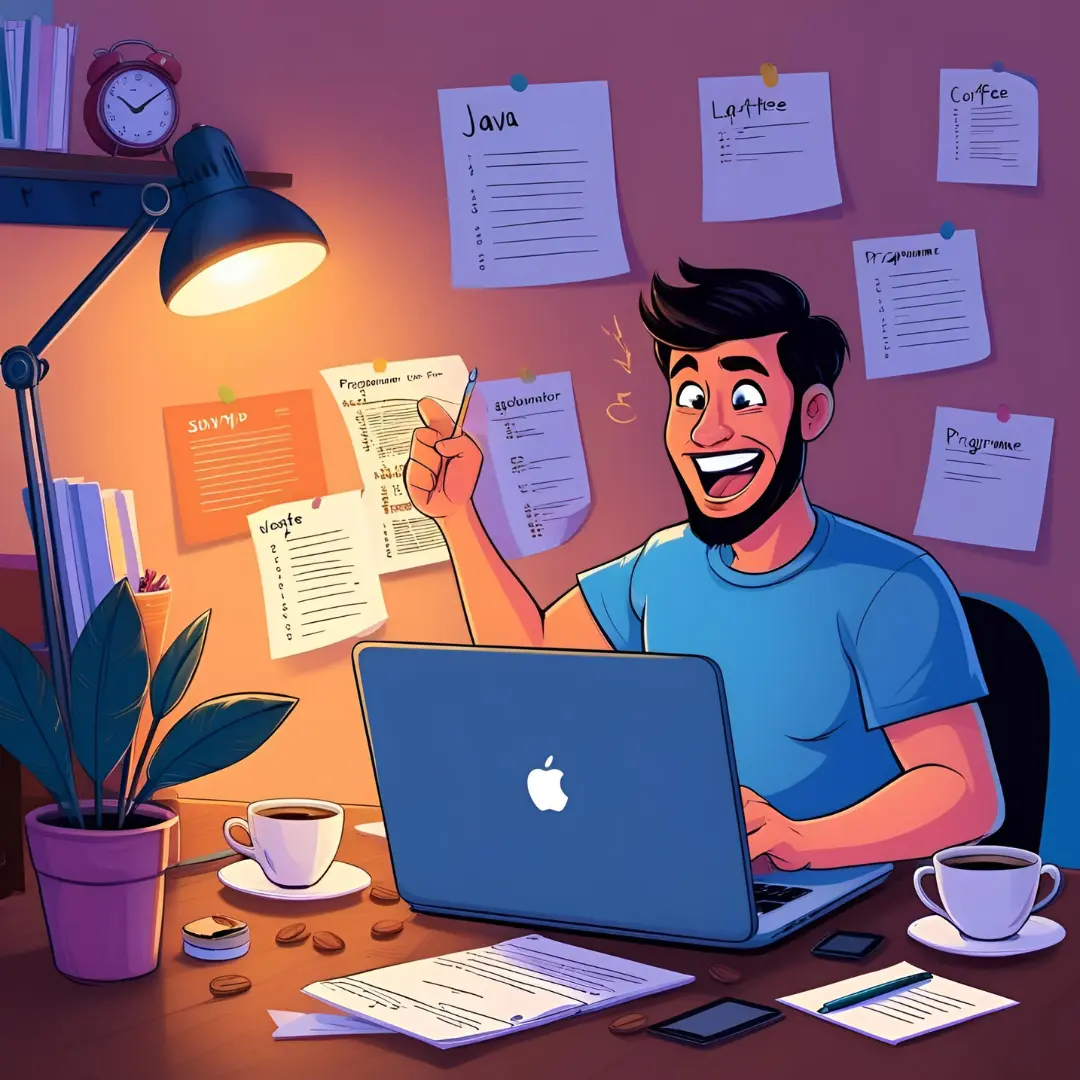
JShell is Java’s Read-Eval-Print Loop (REPL), introduced in Java 9, allowing developers to run Java code interactively without compiling a full program.
1. What is JShell?
✅ Instantly execute Java code – No need to create a full main()
method.
✅ Great for quick testing and learning – Experiment with APIs before using them in projects.
✅ Improves developer productivity – Quickly check logic without setting up a project.
2. How to Start JShell?
🔹 Step 1: Open your terminal or command prompt.
🔹 Step 2: Run JShell:
jshell
🔹 Step 3: Start typing Java code interactively!
✅ Example: Running simple Java commands in JShell
jshell> int x = 5;
x ==> 5
jshell> x + 10
$2 ==> 15
✔ JShell evaluates expressions immediately.
3. Key Features of JShell
Feature | Description | Example |
---|---|---|
Variable Declaration | Assign values to variables | int x = 10; |
Method Definition | Define methods without a class | int square(int n) { return n * n; } |
Class Definition | Define classes interactively | class Person { String name; } |
Auto Imports | No need to import java.util.*; | List<String> list = List.of("A", "B"); |
Multi-line Code | Write multi-line methods and loops | for (int i = 0; i < 5; i++) { System.out.println(i); } |
History Navigation | Use up/down arrows to cycle through past commands | x + 5 (Press ↑ to reuse) |
Tab Completion | Auto-completes class names, methods, and variables | System. (Press Tab) |
4. Running Code in JShell
📌 Example 1: Defining and Using a Variable
jshell> int age = 25;
age ==> 25
jshell> age + 5
$2 ==> 30
✔ JShell automatically evaluates expressions.
📌 Example 2: Writing Methods in JShell
jshell> int square(int n) { return n * n; }
| Created method square(int)
jshell> square(4)
$3 ==> 16
✔ No need to define a class or main()
method!
📌 Example 3: Creating a Simple Class
jshell> class Person {
...> String name;
...> int age;
...> Person(String n, int a) { name = n; age = a; }
...> void display() { System.out.println(name + " is " + age + " years old."); }
...> }
| Created class Person
jshell> Person p = new Person("Alice", 30);
p ==> Person@6a6824be
jshell> p.display()
Alice is 30 years old.
✔ JShell allows defining and using classes dynamically.
5. Useful JShell Commands
Command | Description |
---|---|
/help | Show all JShell commands |
/vars | List all declared variables |
/methods | Show defined methods |
/list | Show command history |
/edit <n> | Edit previous command <n> |
/save filename.jsh | Save the current session to a file |
/open filename.jsh | Load and run a saved session |
/exit | Exit JShell |
✅ Example: Checking all variables
jshell> /vars
age : int = 25
p : Person = Person@6a6824be
6. Using JShell in Real-World Scenarios
✅ Quickly test Java code snippets before adding them to a project
✅ Experiment with Java Streams, Collections, and Lambdas
✅ Debug logic without writing an entire program
📌 Example: Testing Streams API in JShell
jshell> List.of("Alice", "Bob", "Charlie").stream()
...> .filter(name -> name.startsWith("A"))
...> .forEach(System.out::println);
Alice
✔ JShell helps quickly verify Java 8+ Stream API behavior.
Lesson Reflection
- How does JShell improve developer productivity compared to writing full Java programs?
- When would you use JShell instead of a traditional Java file?
- Can you think of a scenario where JShell would be useful in debugging?
Answers to Reflection Questions on JShell
1️⃣ How does JShell improve developer productivity compared to writing full Java programs?
✅ Eliminates the Need for Boilerplate Code
- Traditional Java programs require a class, a
main()
method, and imports, even for simple tasks. - JShell allows running Java code instantly without writing a full program.
📌 Example: Testing a Simple Calculation
🔴 Traditional Java Approach (Full Class & Main Method)
public class Main {
public static void main(String[] args) {
int result = 10 * 5;
System.out.println(result);
}
}
✅ JShell Approach (No Setup Needed!)
jshell> 10 * 5
$1 ==> 50
🚀 JShell executes it instantly—no need for a full program!
2️⃣ When would you use JShell instead of a traditional Java file?
✅ 1. When testing small Java snippets quickly
- Instead of creating a full project, you can test methods, loops, and expressions interactively.
✅ 2. When learning Java APIs or new features
- If you want to explore Java Streams, Optional, or new Java features, JShell is the fastest way to test them.
📌 Example: Testing Streams in JShell
jshell> List.of("Alice", "Bob", "Charlie").stream()
...> .map(String::toUpperCase)
...> .forEach(System.out::println);
ALICE
BOB
CHARLIE
✔ JShell lets you experiment with Java Streams in real time!
✅ 3. When debugging logic without recompiling code
- JShell remembers previous variables and methods, so you can test fixes instantly.
3️⃣ Can you think of a scenario where JShell would be useful in debugging?
🚀 Scenario: Debugging a Method Without Running the Full Application
📌 Problem:
A developer is working on a method to reverse a string but isn’t sure if it works correctly.
🔴 Traditional Debugging Approach:
- Edit code → Compile → Run → Print debug output → Repeat if needed.
✅ JShell Debugging Approach:
jshell> String reverse(String str) { return new StringBuilder(str).reverse().toString(); }
| Created method reverse(String)
jshell> reverse("hello")
$2 ==> "olleh"
✔ JShell helps test the method immediately—no compilation needed!
🔍 Key Takeaways
✔ JShell removes boilerplate code and allows quick Java execution.
✔ JShell is useful for testing, learning Java APIs, and debugging code interactively.
✔ It is best used for quick experiments, but full Java files are still needed for real-world applications.
The next Java 10+ feature: Local Variable Type Inference (var
)? 😊🚀