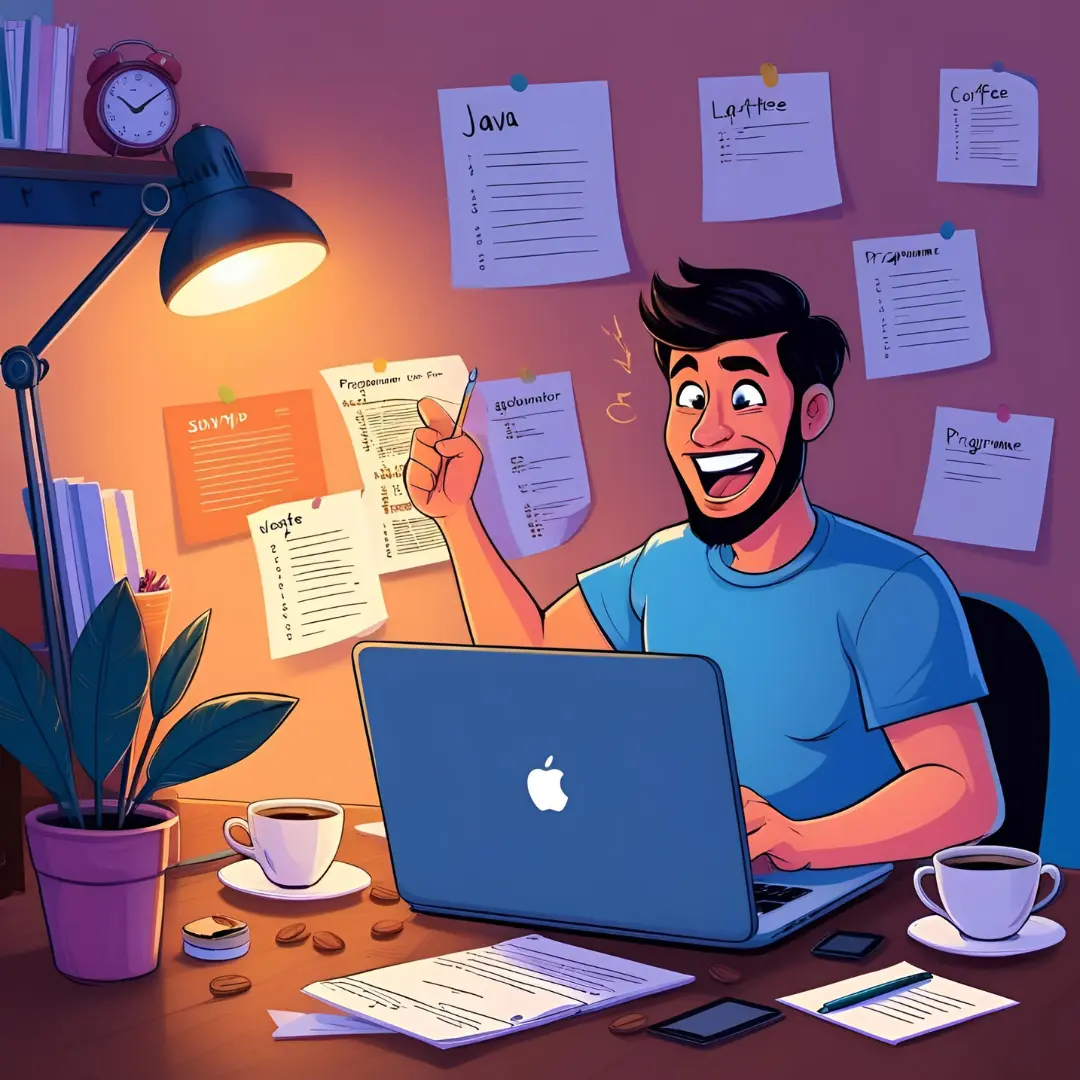
What is a Lambda Expression?
A Lambda Expression is a shorthand way to define anonymous functions (functions without a name). It allows you to write cleaner, more readable code by reducing boilerplate when using functional interfaces.
Syntax of a Lambda Expression
(parameters) -> { body }
Example 1: Without Lambda (Before Java 8)
interface Greeting {
void sayHello();
}
public class Main {
public static void main(String[] args) {
Greeting greeting = new Greeting() {
@Override
public void sayHello() {
System.out.println("Hello, Java 8!");
}
};
greeting.sayHello();
}
}
Example 2: With Lambda (Java 8)
interface Greeting {
void sayHello();
}
public class Main {
public static void main(String[] args) {
Greeting greeting = () -> System.out.println("Hello, Java 8!");
greeting.sayHello();
}
}
Why is this better?
- Less Code – No need for anonymous inner classes.
- More Readable – Code is shorter and easier to understand.
Lesson Task
- Write a lambda expression for a functional interface that takes a number and prints whether it’s even or odd.
- Try running both traditional and lambda-based implementations.
Question:
Can lambda expression be used for both interface and regular classes?
Answer:
Lambda expressions in Java can only be used with functional interfaces, not with regular classes.
Why Only Functional Interfaces?
A functional interface is an interface with exactly one abstract method. Lambda expressions provide a way to implement that single method without explicitly creating an anonymous class.
Example of a Functional Interface (Works with Lambda)
@FunctionalInterface
interface Greeting {
void sayHello();
}
public class Main {
public static void main(String[] args) {
Greeting greeting = () -> System.out.println("Hello, Lambda!");
greeting.sayHello();
}
}
Here, Greeting
has only one abstract method (sayHello
), so we can use a lambda expression to implement it.
Why Can’t We Use Lambda with Regular Classes?
A regular class can have multiple methods, so a lambda expression would not know which method to implement. Lambdas are designed to work with single-method interfaces where the implementation is clear.
Example: Cannot Use Lambda for Regular Class
class RegularClass {
void method1() { System.out.println("Method 1"); }
void method2() { System.out.println("Method 2"); }
}
// ❌ ERROR: Cannot use lambda because RegularClass is not a functional interface
RegularClass obj = () -> System.out.println("Hello");
This would result in a compilation error because RegularClass
has multiple methods, and Java cannot determine which one the lambda should implement.
How to Work Around This?
If you want to use lambdas with a class-like structure, you have two options:
- Use Functional Interfaces
- If your class is designed to have only one behavior, make it a functional interface.
- Use Anonymous Classes for Multi-Method Classes
- If you need multiple methods, use anonymous inner classes instead.
Key Takeaways
- ✅ Lambda expressions only work with functional interfaces (interfaces with a single abstract method).
- ❌ Lambda expressions cannot be used to instantiate regular classes, even if they have only one method.
- ✅ For regular classes, use anonymous classes or create a functional interface instead.
next topic, Functional Interfaces.