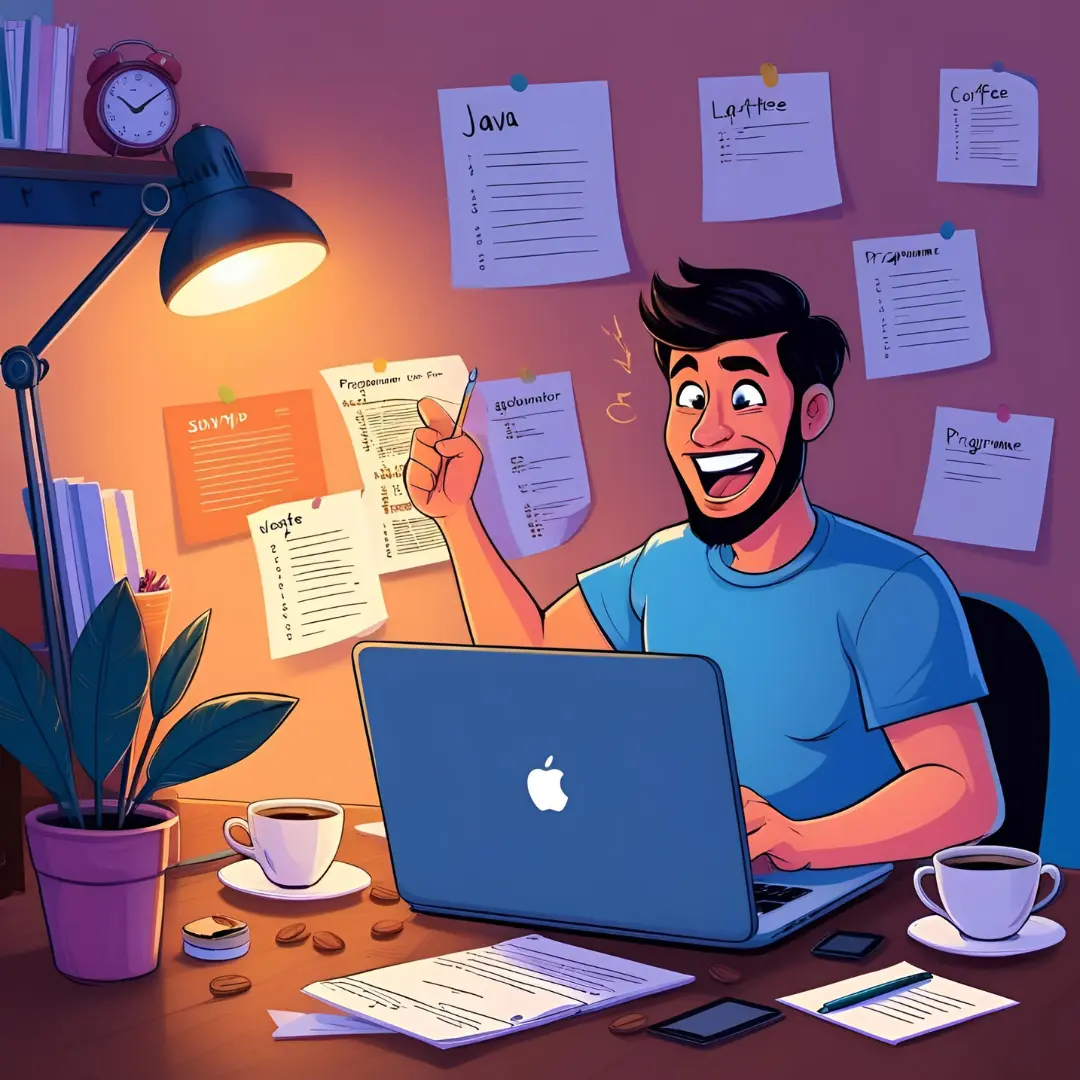
โ Java Feature Cheat Sheet
๐ง Java 8 (2014) โ The Functional Revolution
- ๐น Lambda Expressions โ
(x) -> x * 2
- ๐น Streams API โ
list.stream().filter(...).collect(...)
- ๐น Functional Interfaces โ
Predicate<T>
,Function<T, R>
, etc. - ๐น Default Methods in interfaces
- ๐น Optional<T> to avoid
null
๐ฆ Java 9 (2017) โ Modularity & Interactivity
- ๐น Module System โ
module-info.java
- ๐น JShell โ Java REPL (
jshell
) - ๐น Factory Methods for Collections โ
List.of()
,Set.of()
,Map.of()
๐ฉ Java 10 (2018) โ Simplified Syntax
- ๐น
var
keyword โ Local variable type inference
๐จ Java 11 (2018) โ Practical Utilities
- ๐น New String methods:
strip()
,lines()
,repeat()
- ๐น New File methods:
Files.readString()
,Files.writeString()
๐ช Java 12 (2019) โ Functional Stream Enhancements
- ๐น
Collectors.teeing()
โ Process a stream in two collectors at once
๐ซ Java 13 (2019) โ Multiline Strings
- ๐น Text Blocks โ
""" multiline """
strings
๐ฅ Java 14 (2020) โ Smarter Type Checks
- ๐น Pattern Matching for
instanceof
โ No more casting needed:if (obj instanceof String s)
๐ฆ Java 15 (2020) โ Secure Inheritance
- ๐น Sealed Classes โ
Limit which classes can extend a base classsealed class A permits B, C {}
๐ฉ Java 16 (2021) โ Immutable Simplicity
- ๐น Records โ
Auto-generate immutable data classesrecord Person(String name, int age)
๐จ Java 17 (2021 LTS) โ Modern Switch
- ๐น Pattern Matching in
switch
- ๐น Enhanced Sealed Types
- ๐น Strong Encapsulation for JDK Internals
๐ง Java 19 (2022) โ Concurrency Breakthrough
- ๐น Virtual Threads (Preview)
โ Lightweight threads for massive concurrencyThread.ofVirtual().start(...)
๐ฆ Java 21 (2023 LTS) โ Modern Java Matures
- ๐น String Templates (Preview) โ
STR."Hello, \{name}!"
- ๐น Scoped Values โ Safe replacement for
ThreadLocal
- ๐น Sequenced Collections โ
getFirst()
,getLast()
,reversed()
onList
,Set
,Map
- ๐น Structured Concurrency โ
StructuredTaskScope
for managing parallel tasks - ๐น Unnamed Variables & Patterns โ
_
to ignore values cleanly
๐ฅ Java 22 (2024) โ Native Interop & Pattern Power
- ๐น Foreign Function & Memory API (Preview)
โ Replace JNI, call C functions, manage native memory - ๐น Enhanced Pattern Matching &
_
Usage
โ Use_
ininstanceof
,switch
, and nested patterns - ๐น More switch power:
case _ ->
now allowed with patterns
๐งพ Tip: Use Java 21 as your default LTS baseline
It brings modern programming style, cleaner concurrency, better formatting, and structured error handling.