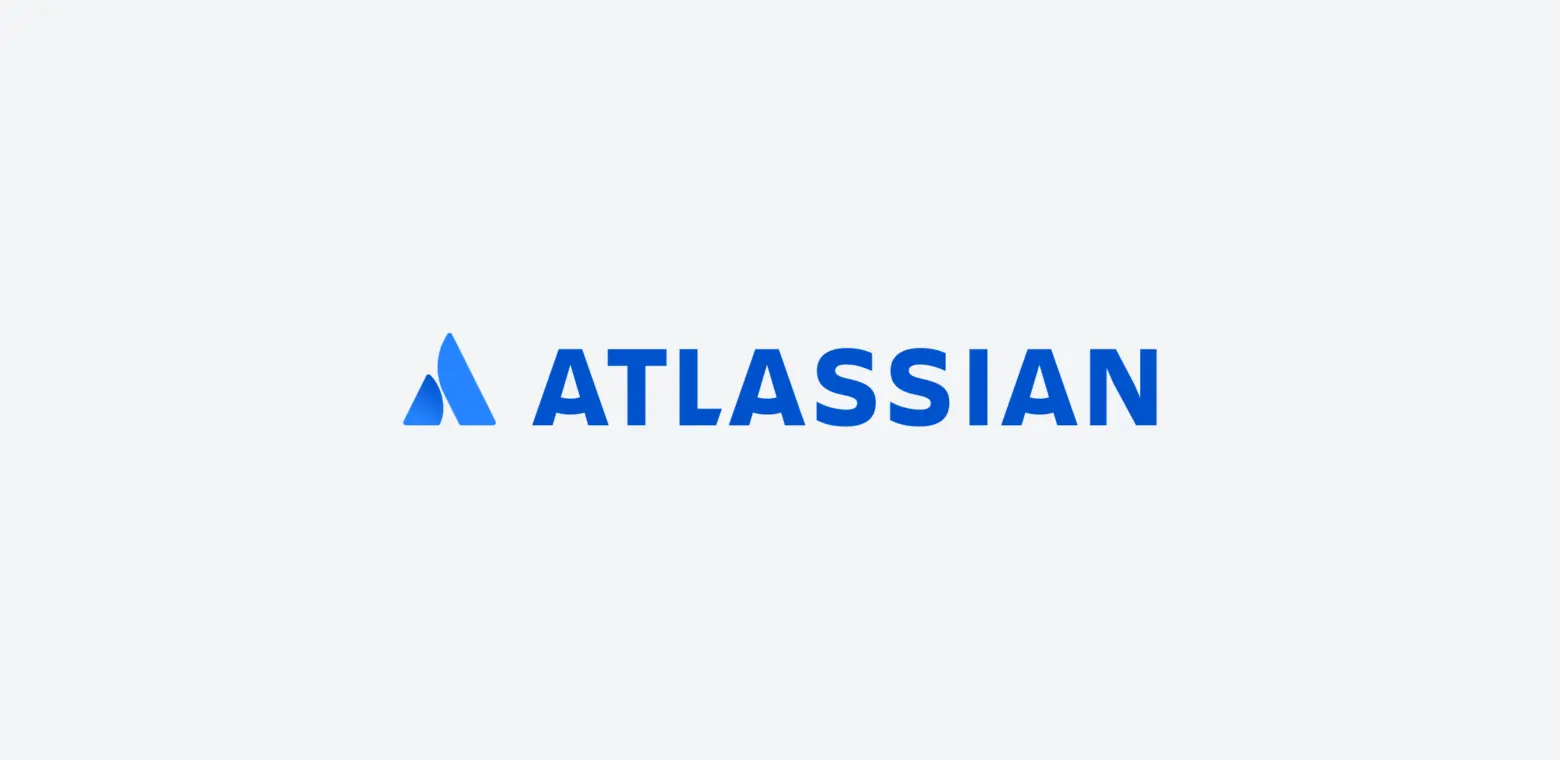
🧭 Overview of Atlassian’s Interview Process
✅ Stage 1: Recruiter Screen
- What it’s about:
- Resume & background discussion
- Overview of the interview process
- High-level technical questions (maybe)
- Salary expectations & notice period
Tip: Be familiar with Atlassian’s mission and values.
💻 Stage 2: Online Coding Assessment (HackerRank or Karat)
- Duration: 60–90 minutes
- Focus: Data structures and algorithms
- Format:
- 1–2 coding problems (Leetcode medium)
- Time complexity matters
- Tests edge cases and correctness
Tip: Practice on Leetcode, especially problems involving arrays, hash maps, strings, trees, and graphs.
🧑💻 Stage 3: Technical Interview / Coding Round
- Format: Live coding on a shared editor (Zoom, Karat, or CodePair)
- What they assess:
- Problem-solving approach
- Clean, working code
- Edge cases and test cases
- Communication while coding
Example questions:
- Reverse a linked list
- Detect cycles in a graph
- Design a rate limiter
- String compression algorithms
🏗️ Stage 4: System Design Round (Mid-level and up)
- What to expect:
- High-level and low-level system design
- API design, data storage, scaling
- Trade-offs and justifications
Example prompts:
- Design a simplified version of Jira/Trello
- Design a notification system
- Design an audit logging service
Tip: Be clear on things like:
- Component diagrams
- Caching strategies
- Read/write patterns
- CAP theorem trade-offs
🎯 Stage 5: Behavioral Interview (Values Fit / Leadership Principles)
- Atlassian takes culture seriously.
- Interviewer may be a manager, peer, or someone from another team.
Topics:
- A time you gave/received feedback
- Handling conflict or failure
- Working cross-functionally
- Aligning with Atlassian’s values (e.g. “Be the change you seek”)
Use the STAR format: Situation → Task → Action → Result
⚙️ Optional: Technical Deep Dive / Past Project
- Especially for senior candidates
- Discuss a past project in detail
- Justify design decisions, challenges, trade-offs
🧠 Atlassian Core Values (They often ask behavioral questions around these)
- Open company, no bullshit
- Build with heart and balance
- Don’t #@!% the customer
- Play, as a team
- Be the change you seek
Be ready to show how you’ve lived these values in your work.
📌 Final Thoughts & Tips
- Clarity matters – Always explain your approach before coding.
- Test your code – Ask for input/output or walk through it.
- Culture fit is key – They value empathy, communication, and team collaboration.
- Use examples – In behavioral rounds, real stories show authenticity.
- Be yourself – They’re known for a humble, collaborative engineering culture.
Here’s a breakdown each stage in the Atlassian Software Engineer interview process, with insights, preparation tips, and red flags to avoid.
✅ Stage 1: Recruiter Screen
🎯 Purpose:
- Gauge basic fit, experience, communication skills, and alignment with Atlassian’s values.
- Explain the full process and timeline.
🧠 What to Prepare:
- Your elevator pitch – 30–60 second summary of your background and interests.
- Why Atlassian? Why this role?
- High-level overview of your current/past projects.
- Be familiar with their products (Jira, Confluence, Trello, etc.)
- Know their core values (they really care about these!)
⚠️ Watch Out:
- Don’t give vague answers about your role or responsibilities.
- Don’t undersell your achievements.
- If you’re unenthusiastic or unprepared, it’s a red flag.
💻 Stage 2: Online Coding Assessment (HackerRank or Karat)
🎯 Purpose:
- Assess your problem-solving and coding fundamentals under time pressure.
🧠 What to Expect:
- Platform: HackerRank or Karat (live)
- Duration: 60–90 minutes
- 1–2 coding problems (Leetcode easy-medium-hard)
- Edge cases & performance matter
🧪 Topics:
- Strings, arrays, hashmaps, recursion
- Sorting, searching
- Linked lists, trees, graphs (BFS/DFS)
- Sliding window, two pointers
- Time/space complexity
✅ What To Do:
- Think out loud (if live)
- Write clean, readable code
- Add meaningful variable names
- Test with custom test cases
⚠️ Watch Out:
- Don’t skip writing test cases.
- Don’t get stuck in one approach – switch if you’re blocked.
- Avoid brute force if possible – optimize if you have time.
🧑💻 Stage 3: Technical Interview / Live Coding Round
🎯 Purpose:
- Test your real-time problem-solving skills, communication, and coding under observation.
🧠 What To Expect:
- Pairing-style coding over a shared IDE (CoderPad, CodeSignal, Karat, or Zoom)
- May involve 1 or 2 coding questions
- Expect follow-up: optimizing your solution or adapting it to new constraints
✅ Tips:
- Clarify the problem out loud.
- Ask questions if anything is ambiguous.
- Use examples to walk through your logic.
- Verbally walk through your plan before coding.
- After writing code, walk through edge cases.
⚠️ Watch Out:
- Jumping into code without planning = 🚩
- Not handling edge cases = 🚩
- Poor communication / lack of collaboration = 🚩
🏗️ Stage 4: System Design Interview
Usually for mid-level and above (SDE II and higher). Juniors may get a simplified design question.
🎯 Purpose:
- Evaluate your ability to design scalable, maintainable systems.
- Assess how you handle trade-offs and structure software architecture.
🧠 What To Expect:
- 45–60 mins
- “Design Jira Ticketing System”, “Notification System”, “Rate Limiter”, etc.
- Expect follow-ups like: “How would you scale it?”, “How would you handle X failure?”, “How would you test it?”
Topics You Should Know:
- API Design (RESTful)
- Databases (SQL vs NoSQL, indexing, normalization)
- Caching (Redis, CDN)
- Load balancing, rate limiting
- Asynchronous processing (queues, pub/sub)
- Fault tolerance, replication
✅ Tips:
- Start high-level (draw or describe architecture)
- Talk about each component: web, backend, DB, caching, etc.
- Consider scalability, availability, and data consistency
- Think through failure points and monitoring
⚠️ Watch Out:
- Ignoring edge cases like failure, latency, or downtime
- Jumping into low-level details too early
- Forgetting API versioning, rate limiting, or auth
🎯 Stage 5: Behavioral / Culture Fit Interview
🎯 Purpose:
- See if you’ll thrive in Atlassian’s team-first, feedback-positive environment.
- Gauge alignment with their core values.
🧠 Common Questions:
- “Tell me about a time you disagreed with a teammate.”
- “How do you handle feedback?”
- “Tell me about a failure and what you learned.”
- “Describe a time you improved a process or tool.”
✅ Tips:
- Use STAR format (Situation, Task, Action, Result)
- Be honest and reflective
- Show empathy, growth mindset, and collaboration
- Know Atlassian’s values and connect your answers to them
⚠️ Watch Out:
- Giving generic answers with no real story
- Blaming others in failure stories
- Showing lack of initiative or ownership
⚙️ Optional: Technical Deep Dive / Project Discussion
More common for mid/senior-level roles
🎯 Purpose:
- Dive deep into your past experience
- Assess architectural thinking, impact, technical depth
🧠 What To Prepare:
- 1–2 key projects you’ve worked on (ideally with architectural/design elements)
- Explain the problem, how you solved it, challenges, and the impact
- Be ready to discuss trade-offs (e.g., why Kafka vs RabbitMQ, why SQL vs NoSQL, etc.)
✅ Tips:
- Make it relevant to the role
- Use diagrams or analogies to explain complex ideas
- Highlight impact (e.g., reduced latency by X%, improved throughput by Y%)
📋 Summary Cheat Sheet
Stage | Focus | What to Nail | Red Flags |
---|---|---|---|
Recruiter Screen | Fit + Culture | Enthusiasm, background | Vagueness, low energy |
Coding Challenge | DSA | Clean code, edge cases | No tests, brute force |
Live Coding | Problem solving | Communication, reasoning | Jumping to code blindly |
System Design | Architecture | High-level to low-level design | Missed trade-offs, no scaling |
Behavioral | Culture fit | Real stories, STAR method | Generic or blaming answers |
Project Deep Dive | Technical depth | Clear explanation, impact | Lack of ownership |