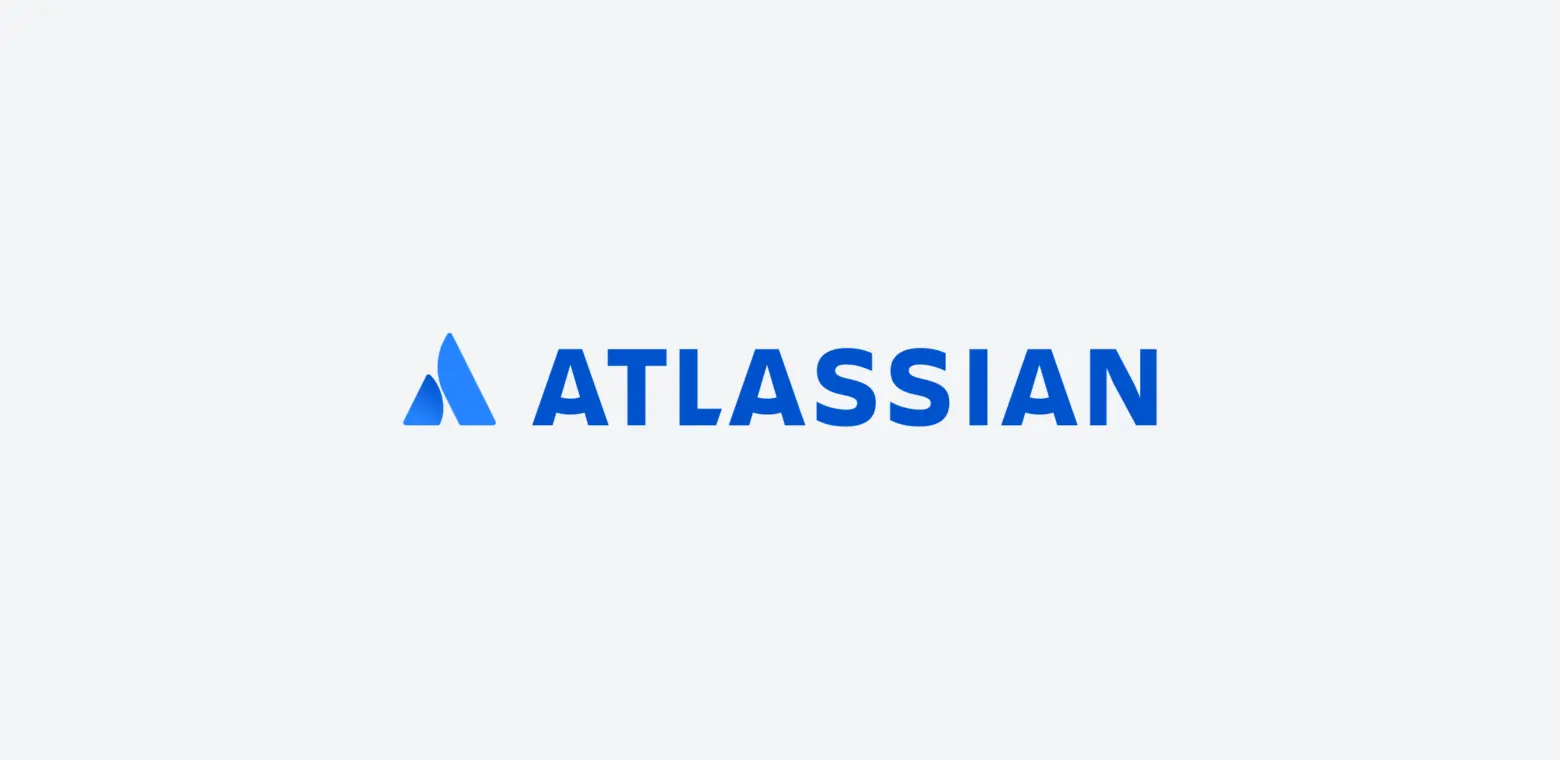
🧩 Mock System Design Prompt:
“Design a Ticketing System like Jira”
🎯 Prompt:
Design a simplified version of Jira where users can create, view, assign, and update tickets within projects. Your system should support:
- Projects with multiple users
- Tickets (issues) with title, description, status, assignee, comments
- Ticket status:
To Do
,In Progress
,Done
- Users can filter/search tickets by project, status, or assignee
- System should be scalable to millions of users and projects
✅ What the Interviewer is Evaluating
- Can you model data and flows clearly?
- Do you understand system components and communication?
- How do you handle scalability, consistency, and fault tolerance?
- Do you think about API design, data modeling, rate limiting, etc.?
🧠 What You Should Do (Live or Whiteboard)
1. Clarify the Scope
Ask clarifying questions:
- Is this just internal Jira for a company, or public SaaS?
- How real-time does it need to be (e.g. notifications/comments)?
- Do we need user authentication?
- Are we designing for MVP or full production?
2. Define Core Entities
Basic models:
- User:
user_id
,name
,email
, etc. - Project:
project_id
,name
,owner_id
,members
- Ticket:
ticket_id
,title
,description
,status
,created_at
,updated_at
,assignee_id
,project_id
- Comment:
comment_id
,ticket_id
,user_id
,text
,timestamp
Use a relational DB (PostgreSQL) for core data and add ElasticSearch for full-text search.
3. High-Level System Components
- API Gateway
- Authentication Service (OAuth, JWT)
- Project Service
- Ticket Service
- Search Service
- Comment Service
- Notification Service (optional)
- Database(s) – Primary (Postgres/MySQL), Secondary (Elastic, Redis)
- Object Storage (for attachments, optional)
4. Design Key APIs
Examples:
POST /projects/{id}/tickets
GET /projects/{id}/tickets?status=todo&assignee=123
PATCH /tickets/{ticket_id}
POST /tickets/{ticket_id}/comments
5. Database Schema (Simplified)
Use normalized SQL tables for data integrity:
users
,projects
,project_members
,tickets
,comments
Indexes:
- Index on
project_id
,status
,assignee_id
intickets
- Full-text index for title/description via ElasticSearch
6. Scale & Reliability
- Read-heavy workload → Use read replicas
- Horizontal scaling of stateless services (ticket, project, comment)
- Use Redis or Memcached to cache hot queries (e.g. most viewed tickets)
- Rate limiting to prevent abuse (API Gateway)
- Monitoring + Logging (Prometheus + Grafana + ELK)
7. Advanced Considerations
- Notifications: via WebSocket or Push (e.g. “comment added”)
- Audit Logs: who changed what & when
- Tagging or labels on tickets
- Activity Feed per project/user
- Analytics for project velocity, ticket cycle time, etc.
- Pagination and filtering for scalability
8. Bottlenecks & Trade-Offs
- Search: Do we need real-time indexing? Eventual consistency?
- Scaling comments: Separate service with pagination
- Data Consistency vs. Performance: Strong in core tables, eventual in search
- Write spikes (e.g. mass ticket creation): Queue/batch processing
🎤 Wrap-Up
Before finishing, summarize:
- Your high-level architecture
- How you would improve it over time
- How it handles growth and failures
- Optional features you’d add (attachments, audit trail, kanban view, etc.)
Follow up questions and answers:
🎤 Interviewer:
1. What are the core features and components you’d include in your MVP for this ticketing system?
✅ Candidate:
For the MVP of a simplified Jira-like system, I’d include the following core features:
🔧 Core Functionalities:
- User Management – Create/manage user accounts (can be stubbed with a basic auth system initially).
- Project Management – Users can create projects and invite others.
- Ticketing System – Create, assign, update, and delete tickets.
- Ticket Comments – Add/view comments on a ticket.
- Basic Filtering/Search – Filter tickets by project, status, or assignee.
- Activity Timestamps – Track created/updated times.
🧱 High-Level Components:
- API Gateway
- Authentication Service (OAuth or JWT)
- User Service
- Project Service
- Ticket Service
- Comment Service
- Search Service (for filtering/searching tickets)
- PostgreSQL for core data
- ElasticSearch for advanced search
- Redis for caching ticket lookups
The MVP focuses on collaboration and tracking workflows. Real-time features, permissions, notifications, and analytics can be added later.
🎤 Interviewer:
2. How would you model the ticket entity in your database? What indexes would you add for performance?
✅ Candidate:
I’d use a relational database like PostgreSQL for transactional integrity and schema enforcement.
🎯 Ticket Table Schema:
tickets (
ticket_id UUID PRIMARY KEY,
project_id UUID,
title TEXT,
description TEXT,
status ENUM('todo', 'in_progress', 'done'),
assignee_id UUID,
created_at TIMESTAMP,
updated_at TIMESTAMP
)
📌 Indexes:
(project_id)
— to filter by project(assignee_id)
— for assigned ticket filtering(project_id, status)
— for filtering status per project- Full-text index on
title
anddescription
using ElasticSearch for search functionality
This structure allows efficient querying and is horizontally scalable through project-level partitioning if needed.
🎤 Interviewer:
3. How would you scale the system to support millions of tickets and users across many teams?
✅ Candidate:
To scale the system, I’d break it down across these dimensions:
⚙️ Horizontally Scalable Services:
- Each service (user, ticket, project, comment) would be stateless and deployed independently using containers (e.g., Docker + Kubernetes).
- Use load balancers (e.g., NGINX or AWS ALB) in front of service replicas.
🗃️ Database Scaling:
- Sharding by project ID or organization ID to avoid single-node bottlenecks.
- Read replicas for read-heavy operations (e.g., ticket listing).
- Use Redis for caching hot tickets or common filter results.
📡 Search Scalability:
- ElasticSearch cluster for full-text indexing, sharded by project or region.
⏳ Asynchronous Jobs:
- Use message queues (e.g., Kafka or RabbitMQ) for non-critical tasks like sending notifications or indexing search data.
🔐 Security & Isolation:
- Tenant-aware logic to isolate data access per team or organization.
With this approach, the system could scale horizontally to millions of users and high ticket volume while remaining performant and maintainable.
🎤 Interviewer:
4. Let’s say a user posts a comment on a ticket. What happens in the system from request to persistence? How would you keep the user experience smooth?
✅ Candidate:
When a user posts a comment, the system flow would be:
🛠️ Request Flow:
- The frontend sends a
POST /tickets/{id}/comments
request with the comment text. - The API Gateway authenticates the request (JWT).
- It routes to the Comment Service.
- The Comment Service:
- Validates the ticket exists
- Writes the comment to the
comments
table in the DB - Adds a timestamp and user info
- Publishes an event to a message queue like Kafka
- A background worker listens for comment events:
- Triggers notifications (e.g., WebSocket update or email)
- Updates the ticket’s “last updated” field
- API responds with the newly created comment to the user
✨ Smooth UX Considerations:
- Optimistic UI update: Show the comment on the frontend immediately while the backend persists it.
- Retry strategy: Handle network failures gracefully.
- Caching: Use Redis to cache recent comments for fast loading.
This event-driven architecture decouples the core logic from secondary tasks and keeps user experience snappy.